ColdFusion Like JavaScript Functions
Oct 5 |
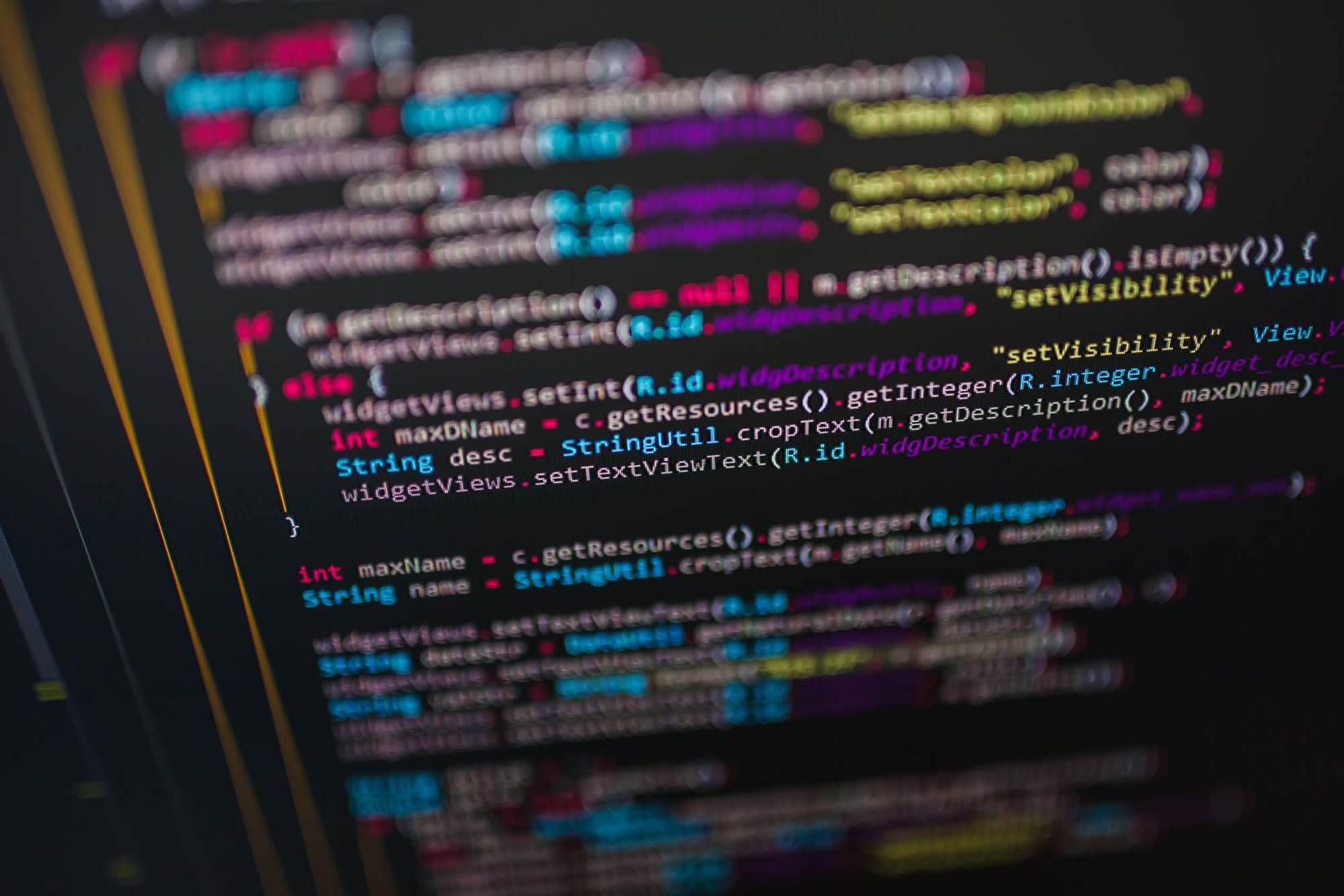
While writing an article with complex JavaScript for my next Kendo UI article, I noticed that I used some ColdFusion-like JavaScript functions to compare comma-separated lists and realized I should first share the code. I don't want to confuse my readers and have them wonder where this JavaScript function came from or confuse them by thinking that a snippet may be ColdFusion code.
I have collected these ColdFusion-like JavaScript functions over the last 20 years. I am unsure where some of these originated- or if I wrote them myself. Some of these scripts may have originated from an old repository of JavaScripts tailored for the ColdFusion community, but I have not found this source for the last several years. I wrote or modified many of these scripts, some based on other ColdFusion custom functions, and I have tried my best to recognize the source.
If you have any functions to add or suggest revisions, please let me know!
Table of Contents
- Appending Values to a Form
- replaceNoCase JavaScript Function
- listLen JavaScript function
- listGetAt JavaScript Function
- listFind JavaScript Function
- ListAppend JavaScript Function
- listDeleteValue JavaScript function
- MyDump JavaScript function that replicates the cfdump ColdFusion function
- Further Reading:
Appending Values to a Form
Although this is not similar to an existing ColdFusion function per se- I use it a lot to create and store lists inside hidden forms. I generally use this when looping through data on the client and appending new values to the form.
When the form is posted, I pass the values inside of the form to ColdFusion for server-side processing. The last delimiter argument is optional.
/* This function appends a value to a specified element in the DOM. Delimiter is optional and defaults to a comma */
function appendValueToElement(value,elementId,delimiter){
// Written by Gregory Alexander
// The delimiter is optional and defaults to a comma
if (delimiter == null){
var delimiter = ",";
}
// Get the element
el = $("#" + elementId);
// Does the value contain anything?
if (el.val().length > 0){
// Append the new value to the existing form
el.val(el.val() + delimiter + value);
} else {
// Insert the value into the empty form
el.val(value);
}
}
replaceNoCase JavaScript Function
I wrote this to replicate the replaceNoCase ColdFusion function. This function replaces the occurrences of substring1 with substring2 in the specified scope and is case-insensitive. The scope is either 'one' or 'all' and defaults to one if not supplied.
If you want to use a case-sensitive replace function, remove the 'i' flag
Example: replaceNoCase('ColdFusion is the best server-side language','ColdFusion','Lucee', 'all')
This will substitute Lucee for ColdFusion in the string 'ColdFusion is the best server-side language' and return 'Lucee is the best server-side language'.
I am not trying to degrade ACF in any way (I don't yet use Lucee), but hopefully, the Lucee fans may get a kick out of this!
// Gregory Alexander <www.gregoryalexander.com>
function replaceNoCase(string, subString, replacement, scope){
if(scope == null) { scope = 'one'; }
if (scope == 'all'){
// i is a RegEx ignore case flag, g is global flag
var regEx = new RegExp(subString, "ig");
} else {
// i is an RegEx ignore case flag
var regEx = new RegExp(subString, "i");
}
// i is an ignore case flag, g is global flag
var regEx = new RegExp(subString, "ig");
var result = string.replace(regEx, replacement);
return result;
}
listLen JavaScript function
This is a simple function that provides the length of a list and replicates the listLen ColdFusion function.
Usage: listLen(list [, delimiters ])
Example: listLen('Mount Rainier National Park,Olympic National Park,North Cascades National Park');
This will return the numeric value of 3, representing the number of National Parks in Washington State.
function listLen(list, delimiter){
// Gregory Alexander <www.gregoryalexander.com>
if(delimiter == null) { delimiter = ','; }
var thisLen = list.split(delimiter);
return thisLen.length;
}
listGetAt JavaScript Function
Gets a list value found at a certain position in a list. This should be identical to the ColdFusion listGetAt function.
Usage: listGetAt(list, position [, delimiters])
Example: listGetAt('Arches National Park,Bryce Canyon National Park,Canyonlands National Park,Capitol Reef National Park,Zion National Park', 1, ',');
This will return 'Arches National Park', the first element in the list.
function listGetAt(list, position, delimiter) {
// Gregory Alexander <www.gregoryalexander.com>
if(delimiter == null) { delimiter = ','; }
list = list.split(delimiter);
if(list.length > position) {
return list[position-1];
} else {
return 0;
}
}
listFind JavaScript Function
Like the listFind ColdFusion function, this will return the index position in a list if it finds the value or return zero if nothing was found. I am not sure of the original authorship. The search is case-sensitive.
Usage: ListFind(list, value [, delimiters ])
Example: listFind('1,2,3,4,5,6','5');
This example will return a 5 as it is the 5th index in the list.
function listFind(list, value, delimiter) {
// Adapted from a variety of sources by Gregory Alexander <www.gregoryalexander.com>
var result = 0;
if(delimiter == null) delimiter = ',';
list = list.split(delimiter);
for ( var i = 0; i < list.length; i++ ) {
if ( value == list[i] ) {
result = i + 1;
return result;
}
}
return result;
}
See https://copyprogramming.com/howto/what-is-the-fastest-implementation-of-coldfusion-s-listfindnocase-function-in-javascript for a different approach to this solution.
ListAppend JavaScript Function
This function is identical to the listAppend ColdFusion method. It concatenates a list or element to a list and returns a string.
Usage: listAppend(list, value)
Example: listAppend('Glacier National Park', 'Yellowstone National Park');
This example will append Yellowstone National Park to the list and return 'Glacier National Park', 'Yellowstone National Park' national parks within Montana.
// Adds a value to a comma-separated list. Will not add the value if the list already contains the value.
function listAppend(list, value) {
// Adapted from a variety of sources by Gregory Alexander <www.gregoryalexander.com>
var re = new RegExp('(^|)' + value + '(|$)');
if (!re.test(list)) {
return list + (list.length? ',' : '') + value;
}
return list;
}
listDeleteValue JavaScript function
This JavaScript function deletes a value within a list and is based on Ben Nadel's listDeleteValue function found on GitHub
Usage: listDeleteValue(list, value)
Example: listDeleteValue('Grand Canyon National Park,Saguro National Park,Indian Ocean', 'Indian Ocean');
This will delete 'Indian Ocean' from a list of parks in Arizona and will return 'Grand Canyon National Park,Saguaro National Park.'
// Removes a value in a comma separated list. Based on the ListDeleteValue function by Ben Nadel CF fuction https://gist.github.com/bennadel/9753040
var listDeleteValue = function(list, value){
// Adapted from a variety of sources by Gregory Alexander <www.gregoryalexander.com>
var values = list.split(",");
for(var i = 0 ; i < values.length ; i++) {
if (values[i] == value) {
values.splice(i, 1);
return values.join(",");
}
}
return list;
}
MyDump JavaScript function that replicates the cfdump ColdFusion function
This handy function dumps out a JavaScript object to the console log. It was meant to provide functionality similar to the cfdump tag in ColdFusion.
Usage: mydump(arr, level)
Note: this should be used carefully as it contains a lot of data that could consume many resources.
// function to dump out a a javascript object.
function mydump(arr,level) {
var dumped_text = "";
if(!level) level = 0;
var level_padding = "";
for(var j=0;j<level+1;j++) level_padding += " ";
if(typeof(arr) == 'object') {
for(var item in arr) {
var value = arr[item];
if(typeof(value) == 'object') {
dumped_text += level_padding + "'" + item + "' ...
";
dumped_text += mydump(value,level+1);
} else {
dumped_text += level_padding + "'" + item + "' => "" + value + ""
";
}
}
} else {
dumped_text = "===>"+arr+"<===("+typeof(arr)+")";
}
console.log(dumped_text);
}
Further Reading:
- If you want a more comprehensive library, check out the cfjs project that has replicated 90 ColdFusion functions on GitHub.
- James Molberg has developed a comprehensive tool to replicate the functionality of cfdump. See Javascript version of ColdFusion CFDump
Tags
ColdFusion, JavaScript
![]() |
Gregory Alexander |
Hi, my name is Gregory! I have several degrees in computer graphics and multimedia authoring, and I have been developing enterprise web applications for the last 25 years. I love web technologies and the outdoors and am passionate about giving back to the community. |
This entry was posted on October 5, 2022 at 10:44 PM and has received 1728 views.