Using Cookies to Pass JavaScript Variables to ColdFusion
Jun 16 |
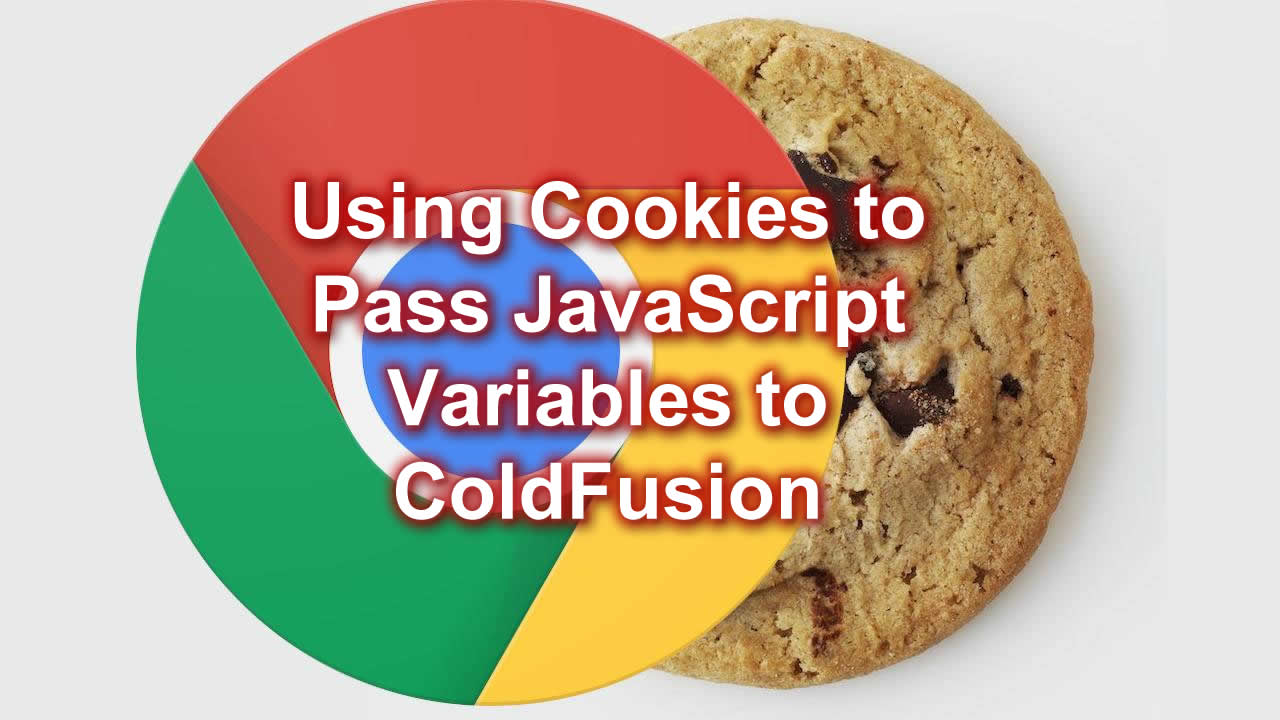
Server-side and client-side technologies are quite different. Client-side logic is processed on the client's computer, whereas server-side logic is processed on the server, and direct communication between these environments is impossible. However, there are many circumstances in which you would want to pass client-side information to the server. In this article, I will share techniques for sending client-side JavaScript variables, such as the browser height and width, to ColdFusion. This technique is not unique to ColdFusion and can be used by any server-side technology.
Table of Contents
Understanding the differences between Javascript and ColdFusion
You can't directly pass a Javascript variable to ColdFusion as the two technologies are quite different.
ColdFusion is processed on the server side before delivering the content to the client. ColdFusion prepares the entire page and renders it to the client before any client-side activity, such as Javascript, can occur.
Javascript is used on the client side, and these variables are only available after ColdFusion initially delivers the HTML to the client.
To pass Javascript variables to ColdFusion, you must use an assistive technology, such as Ajax, or pass the Javascript variables to the server side once the initial page has been rendered. Here, we will focus on using Cookies to transfer information from the client to the server.
Real-world scenario
In Galaxie Blog, I developed a complex create post interface with TinyMce. There are scores of different interfaces depending on the client's screen resolution and device. I could have used media queries and other client-side techniques to build the page, however, the enormous complexity of the various interfaces made it much easier to design these interfaces on the server-side.
To accomplish this, I sniffed the client's device by intercepting the HTTP user agent string with ColdFusion scripts provided by detectmobilebrowers.com. This works flawlessly and detects mobile and desktop devices, but it does not work with newer iPads. Unfortunately, Apple recently removed any identifying strings and made it impossible to detect iPads using the HTTP user agent string. To determine iPad clients, I would have to use Javascript to obtain the screen resolution and pass it to ColdFusion.
Using cookies to pass Javascript variables to ColdFusion
You can use cookies to transfer information from Javascript to ColdFusion. This technique requires the user to land on a page, such as a login form, to obtain the needed information from Javascript.
In my scenario, the user will first hit a login page. The login page has the following Javascript to set a cookie that ColdFusion can read. Note the path argument ('/') at the end of the script. You must store the cookie that Javascript creates in the root directory for ColdFusion to be able to read the cookie.
Logic on the Client Side
/* Cookie functions. The original author of this script is unknown */
function setCookie(name,value,days) {
var expires = "";
if (days) {
var date = new Date();
date.setTime(date.getTime() + (days*24*60*60*1000));
expires = "; expires=" + date.toUTCString();
}
// The path must be stored in the root in order for ColdFusion to read these cookies
document.cookie = name + "=" + (value || "") + expires + "; path=/";
}
Once the user hits the login page, set a cookie using the Javascript function above. We are naming our cookies 'screenWidth' and 'screenHeight' and passing the device's width and height determined by Javascript and keeping the cookie alive for one day.
// Set a cookie indicating the screen size. We are going to use this to determine what interfaces to use when the the screens are narrow.
// (setCookie(name,value,days))
setCookie('screenWidth',$(window).width(),1);
setCookie('screenHeight',$(window).height(),1);
Server-side logic using ColdFusion
To read the cookie using ColdFusion, it is best to use a try block. The syntax for reading the cookie from Javascript differs from the native ColdFusion cookie methods—note the brackets surrounding the cookie name.
<!--- Get client properties. This will be used to set the interfaces depending upon the screen size --->
<cftry>
<cfset screenHeight = cookie['screenHeight']>
<cfset screenWidth = cookie['screenWidth']>
<cfcatch type="any">
<cfset screenHeight = 9999>
<cfset screenWidth = 9999>
</cfcatch>
</cftry>
<!--- Outputting the screen sizes --->
<cfoutput>
screenHeight: #screenHeight#
screenWidth: #screenWidth#
</cfoutput>
You can use this method to transfer any variable obtained by Javascript to ColdFusion as long as the user first hits a landing page.
I may write other articles using other methods, such as Ajax, in the future.
Tags
ColdFusion
![]() |
Gregory Alexander |
Hi, my name is Gregory! I have several degrees in computer graphics and multimedia authoring, and I have been developing enterprise web applications for the last 25 years. I love web technologies and the outdoors and am passionate about giving back to the community. |
This entry was posted on June 16, 2022 at 1:08 AM and has received 1750 views.