Common Hibenate/ColdFusion ORM Errors
Jun 8 |
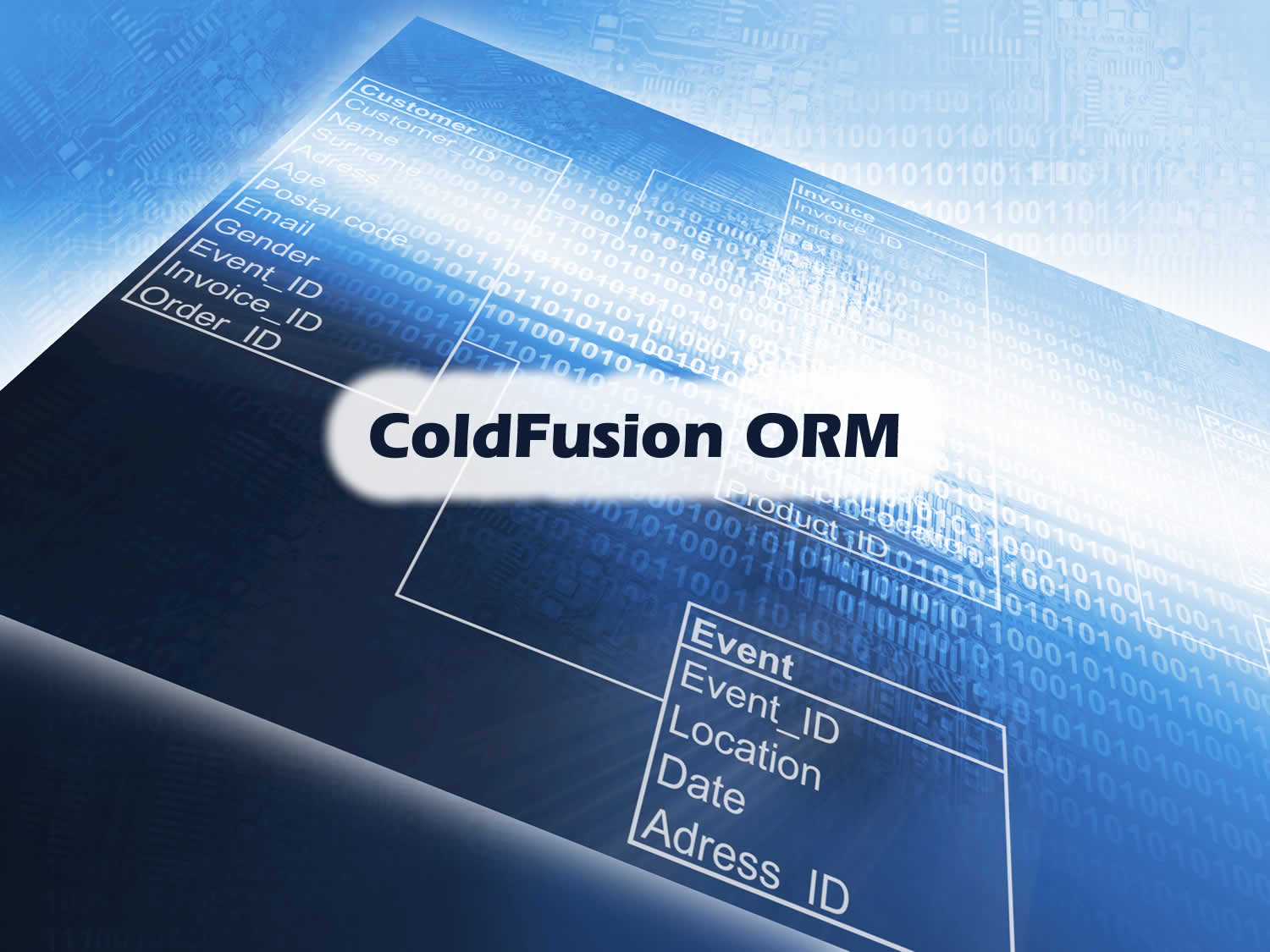
I have worked with ColdFusion ORM for several years now, and one of the most frustrating things working with CF ORM is the lack of friendly error messages. It should be noted that this is not necessarily Adobe's fault- Hibernate, the technology used by ColdFusion provides most of these error messages.
I have compiled a list of common error messages and will try to explain why the error is occurring along with potential resolutions. You may find this useful when developing Java or ColdFusion applications.
Table of Contents
- 500 error with no error message on the page, however, the following error shows up in the ColdFusion application log: coldfusion/orm/hibernate/ConfigurationManager.
- A different object with the same identifier value was already associated with the session
- Another CacheManager with same name 'X' already exists in the same VM
- Attribute linkTable missing from the property
- Cannot load the target CFC
- Cannot invoke "antlr.collections.AST.getText()" because "ident" is null
- Can't cast String [1] to a value of type [Component]
- coldfusion.orm.PersistentTemplateProxy cannot be cast to java.util.Collection to an incompatible type
- Complex object types cannot be converted to simple values and Property true not present in the entity errors
- could not extract ResultSet
- Error casting an object of type to an incompatible type.
- Deleted object would be re-saved by cascade (remove deleted object from associations)
- Element x is undefined in a Java object of type class java.util.HashMap.
- entity [X] does not exist, existing entities are:
- Error casting an object of type java.lang.Integer to an incompatible type
- Error while executing the Hibernate query.
- Error while resolving the relation between CFC common.cfc.db.model.ThemeSetting and CFC common.cfc.db.model.Theme because 'fkcolumn' is specified on both sides.
- expecting CLOSE, found x near line x, column x
- illegal attempt to dereference collection [{synthetic-alias}
- java.sql.SQLException: [Macromedia][SQLServer JDBC Driver][SQLServer]Ambiguous column name 'x'
- X must contain a component or an interface.
- org.hibernate.exception.SQLGrammarException: could not extract ResultSet
- org.hibernate.HibernateException not found by orm
- org.hibernate.hql.internal.ast.QuerySyntaxException: unexpected token: )
- org.hibernate.MappingException: TableX refers to an unmapped class
- org.hibernate.hql.internal.ast.QuerySyntaxException: xxx is not mapped
- org.hibernate.QueryException: No data type for node: org.hibernate.hql.internal.ast.tree.IdentNode
- ORM is not configured for the current application
- Path expected for join!
- Root cause :org.hibernate.HibernateException: Property : x - Object of type class java.lang.String cannot be used as an array
- Session is closed' or 'Session Closed!
- The CacheManager has been shut down. It can no longer be used.
- The system has attempted to use an undefined value, which usually indicates a programming error, either in your code or some system code.
- You have attempted to dereference a scalar variable of type class java.util.ArrayList as a structure with members.
500 error with no error message on the page, however, the following error shows up in the ColdFusion application log: coldfusion/orm/hibernate/ConfigurationManager.
This error may be caused as you have a reserved keyword in one of your persistent cfc's or in the cfclocation set in the application.cfc template.
Generally, this error will be raised before Hibernate is fully loaded and it is not due to any mapping issues. The first thing that I would suggest is to set the globally_quoted_identifiers argument to true: this.ormsettings.hibernate.globally_quoted_identifiers = true. This will escape any special reserved keywords that you may have.
If this does not work, you can also try turning off errors at the ORM level by turning cfc errors off with 'this.ormsettings.skipCFCWithError = true', however, I am not sure if this approach will work. I suspect that the error is raised even before loading any of the persistent cfc's that may cause an error.
In my case, I received this error after upgrading from CF2016 to CF2021 and the error was due to using the reserved keyword 'model' as the folder name that I used to store the persistent cfc's in. However, I did not get any error even with debugging turned on. I had to look into the ColdFusion logs and try to guess where the error came from. Changing the folder from mapping to 'galaxieDb' resolved my particular error thankfully.
A different object with the same identifier value was already associated with the session
This could be caused by several issues, namely:
- This could be due to a unique identifier being duplicated when you save the entity. Check your primary key auto generators to see if the primary key value is duplicated somewhere.
- A well-known Hibernate issue is when the memory is holding relationships between various objects. If you are using Java and Hibernate and your using cascade options, use 'merge' instead of using all.
For ColdFusion, you should eliminate cascade options with all pseudo columns that don't use a one-to-one relationship type. Cascade should not be used with many-to-one or many-to-many relationships according to Adobe.
Another CacheManager with same name 'X' already exists in the same VM
This occurred with Lucee when I changed "update" to "dropcreate" with the ORM settings in the application.cfc template. This may be due to the ORM snapshot that I am using (5.4.29.28)
<cfset this.ormSettings.dbcreate = "dropcreate"><!--- this causes the error --->
<cfset this.ormSettings.dbcreate = "update"><!--- change it back to update to bypass the error --->
Attribute linkTable missing from the property
You need to add a link table to your existing many-to-many relationships.
Cannot load the target CFC
This suggests that there is an unmapped class- check to see if the mapped CFC exists. If it does, check case sensitivity in the name. If that does not solve the issue, use the reloadOrm method. See https://stackoverflow.com/questions/9684736/cf-orm-cannot-load-the-target-cfc for more information.
Cannot invoke "antlr.collections.AST.getText()" because "ident" is null
You may be missing a JOIN in a HQL query- for example, the following fictitious query is missing a join to the permissions table prior to the WHERE clause:
SELECT Users.UserId, Users.User, Permissions.PermissionId FROM Users WHERE UserId = 1 (JOIN Permissions is missing)
Can't cast String [1] to a value of type [Component]
With Lucee, using a default value throws an error as the other entity wants a reference, not the string used in the default parameter.
For example, this works with ACF (note the default="1"):
<cfproperty name="BlogTypeRef" ormtype="int" fieldtype="many-to-one" cfc="BlogType" fkcolumn="BlogTypeRef" cascade="all" missingrowignored="true" hint="Foreign Key to the BlogType.BlogTypeId" default="1">
With Lucee, you can't use default="1" as it references another entity. Instead, omit the default property and use:
<cfproperty name="BlogTypeRef" ormtype="int" fieldtype="many-to-one" cfc="BlogType" fkcolumn="BlogTypeRef" cascade="all" missingrowignored="true" hint="Foreign Key to the BlogType.BlogTypeId">
coldfusion.orm.PersistentTemplateProxy cannot be cast to java.util.Collection to an incompatible type
This may be due to incorrectly mapping a one-to-one relationship when the two entities actually have a many-to-x or x-to-many relationship. This error is reporting that it is expecting an array instead of a single string. Look at the entity's existing one-to-one relationships to find out if there is a missing x-to-many or many-to-x relationship.
Complex object types cannot be converted to simple values and Property true not present in the entity errors
You may be getting these errors when you are trying to make changes to an object that is returning multiple records.
Adobe provides documentation that you may set filters and arguments to load a single record, but I have not gotten this approach to work and had to rewrite my logic to ensure that a single record is always retrieved.
Here is the relevant code to load a unique record from an array found on the Adobe site:
EntityLoad(entityName,[Filter="",unique="",options=""])
I have set the unique argument to true and have used an additional maxresults argument to attempt to load a single record, but this approach failed with the following error message- "Property true not present in the entity"
See below for a list of approaches that I have tried to unsuccessfully use.
<cfset CommenterRefDbObj = entityLoad("Commenter", { Email = arguments.email }, "true", {maxresults=1})> ('Property true not present in the entity.')
<cfset CommenterRefDbObj = entityLoad("Commenter", { Email = arguments.email }, true, {maxresults=1})> ('Property true not present in the entity.')
<cfset CommenterRefDbObj = entityLoad("Commenter", { Email = arguments.email }, unique="true", {maxresults=1})> ('Invalid CFML construct found on line x')
<cfset CommenterRefDbObj = entityLoad("Commenter", { Email = arguments.email }, {unique="true"}, {maxresults=1})> ('Complex object types cannot be converted to simple values.')
And others....
To address this problem, you will have to rewrite your logic to ensure that only a single record exists before loading the entity prior to using its set methods. I have not found a reliable way to pick out the record from an array when using ORM.
could not extract ResultSet
Hibernate error is thrown when a many-to-many join table is not correct. Check your join tables for all relationships that have many-to-many relationships
Error casting an object of type to an incompatible type.
You will receive this error when you're using a link table and using the set methods of other entities when setting nonprimitive value types. This is due to the link table not having relationships mapped in the field type argument.
When you try to create a relationship in a link table that you are trying to create manually, you will receive a 'many-to-many requires a link table'. When you specify a link table in another entity that requires a many-to-many mapping, the link table is automatically assumed as having a many-to-many relationship and you need to leave the fieldtype relationship out. However, since the link table does not have a mapped relationship (ie fieldtype="many-to-many") you can't use objects to populate the columns since there is no mapped relationship. Instead, you must use primitive data types when setting the column value, such as integers.
Deleted object would be re-saved by cascade (remove deleted object from associations)
If you receive this error, you must remove all associations belonging to other tables for this record. For example, I have a many-to-one relationship between a blog category and a blog. Each blog may have multiple categories. However, when I try to delete the category without deleting the reference to the blog, it throws this error.
To delete the category, you must first set the category tables blog reference to null using the Javacast method and then, in a separate transaction, delete the category like so:
Element x is undefined in a Java object of type class java.util.HashMap.
Check to see if you have a null in the database column. Replace the null with an empty string.
entity [X] does not exist, existing entities are:
You may have removed or renamed a persistent ORM-related CFC with which another CFC is associated with. To bypass the error, replace the original CFC.
Error casting an object of type java.lang.Integer to an incompatible type
This could be due to a maddening error when you use 'WHERE 0=0 AND' in your SQL when you use a column that is a foreign key and you're using a cfqueryparam to pass the UserId. For example, the UserRef column in the IpAddress table is a foreign key to the Users.UserId column and the two entity keys have a relationship (many-to-one, one-to-one, etc). The following statement will cause this error:
SELECT new Map (
IpAddress.IpAddress as IpAddress
)
FROM IpAddress as IpAddress
WHERE 0=0
AND UserRef = <cfqueryparam value="#getUserId#" cfsqltype="integer">
To resolve this, simply drop the WHERE 0=0 clause and use the following instead:
SELECT new Map (
IpAddress.IpAddress as IpAddress,
)
FROM IpAddress as IpAddress
WHERE UserRef = <cfqueryparam value="#getUserId#" cfsqltype="integer">
Error while executing the Hibernate query.
org.hibernate.hql.internal.ast.QuerySyntaxException: unexpected AST node
I received this error when I was using a function to use HQL to query the database using a variety of filters such as WHERE Post LIKE 'x' AND Post.Remove = 0, etc. This is probably a bug with the ColdFusion implementation of HQL, however, you can generally fix this issue by limiting your filters to one or two WHERE clauses. If you use Hibernate and not ColdFusion HQL, try setting the nativeQuery argument to true, i.e.: @Query(value = "SELECT Post.PostId FROM Post WHERE Post LIKE '%x'",
nativeQuery = true)
Error while resolving the relation between CFC common.cfc.db.model.ThemeSetting and CFC common.cfc.db.model.Theme because 'fkcolumn' is specified on both sides.
The fkcolumn must be specified on the side whose table has the fkcolumn. On the other side, you must specify mappedby.
expecting CLOSE, found x near line x, column x
You may miss a comma after or before a column, ie SELECT FirstName LastName FROM User. Note the missing comma between the first and last name columns.
illegal attempt to dereference collection [{synthetic-alias}
You may be trying to use a collection, or an array to join two classes without an alias name.
java.sql.SQLException: [Macromedia][SQLServer JDBC Driver][SQLServer]Ambiguous column name 'x'
Properly reference any database columns that may be in two or more tables.
X must contain a component or an interface.
You may have commented out the body in the persistent component cfc.
org.hibernate.exception.SQLGrammarException: could not extract ResultSet
You may have an unknown column or table in your SQL query. Search your stack trace for 'unknown column' or search for 'Caused by: java.sql.SQLSyntaxErrorException' to identify the issue. You also may have a with a join. See https://www.gregoryalexander.com/blog/2022/6/8/common-hibenatecoldfusion-orm-errors#mcetoc_1g53i30jv5i
org.hibernate.HibernateException not found by orm
Another CF2023 error that causes the application to crash. However, there is a ticket and a workaround- see https://tracker.adobe.com/?fbclid=IwAR2D8k_Qc2xUrUJg4IrH0dfSXl34SFO5-Ryh8b_FpqTBlYrKPBloQKfghBA#/view/CF-4218706. Thanks to Mike Hodgson on the Facebook CF Programmers for figuring this one out. I will update this post with more information when it becomes available.
org.hibernate.hql.internal.ast.QuerySyntaxException: unexpected token: )
There is an extra comma at the end of the select statements after the last column. The ')' signifies that you are using a SELECT New Map (col1, col2,) for example.
org.hibernate.MappingException: TableX refers to an unmapped class
There may be some class name issue that is causing a hiccup with hibernate when you have multiple ORM databases.
Generally, the error does not provide which relationship is at fault, so you may have to comment out each relationship (one-to-many and many-to-one) to find out the relationship is causing the error. Don't get locked in and assume the broken relationship, there seems to be a missing class name in the XML that Hibernate generates behind the scenes.
I have only had this error twice during my ORM experience and I am not sure what the cause was. With ColdFusion, I don't know of any way to fix the problem when creating the initial database schema, but I have found that I can remove the relationship during the database creation and successfully add the offending relationship back into the table after the database has been created.
org.hibernate.hql.internal.ast.QuerySyntaxException: xxx is not mapped
Note: xxx refers to the table name
This is an odd error. This can either be because there is no ColdFusion component (cfc) defined with the table name. This could also indicate that the ORM objects are not being loaded on the particular page. Check to see if the table is defined in your database components and also check to see if ORM is being properly initialized for the page.
I encountered this error after updating to CF2023 and applying a workaround for a CF2023-related bug as the components are no longer persisted in the ColdFusion cache. See https://www.greghttps://www.gregoryalexander.com/blog/2023/9/16/Critical-Adobe-ColdFusion-2023-Related-Bugs for more information.
org.hibernate.QueryException: No data type for node: org.hibernate.hql.internal.ast.tree.IdentNode
The column or table name may be misnamed. I received this error when I used the wrong table name in the query. For example, SELECT UserName FROM States- where states should be Users.
ORM is not configured for the current application
- You may have duplicate ORM settings in different parts of your Application.cfc. Generally, you should have the ORM Settings, along with ORMReload(), in the OnApplicationStart in your application.cfc and remove OrmReload in the OnRequestStart (see http://nm1m.blogspot.com/2010/09/working-on-little-app-in-coldfusion-9.html).
- Make sure that ORMReload and InitOrm are not conflicting with each other. You can't have both statements in the same logical block.
If you get a "The passed value does not evaluate to a valid array object" error, it is due to the one-to-many relationship set to the type of an array in the entity declaration.
Path expected for join!
A SQL join was not set correctly.
Root cause :org.hibernate.HibernateException: Property : x - Object of type class java.lang.String cannot be used as an array
The following error is also related to having an incorrect one-to-one relationship when it expects an x-to-many or many-to-x relationship.
Session is closed' or 'Session Closed!
You can't process two or more different database sessions at the same time if you leave the default ORM settings as they are. If you are getting session errors with ORM, change the ORM settings to flushAtRequestEnd=false (<cfset this.ormsettings.flushAtRequestEnd = false>).
After setting this, you must change your code to use cftransactions around all of your ORM operations in order to flush the results unless you choose to manually use flush to maintain your ORM sessions programmatically.
Another issue may be that there are errors in your CRUD operations code, especially if your crud operations are within a loop. What may be going on is that the error is trying to be reported, however, the ORM session that caused the code can't report the error internally as a new ORM session is being created and the original session is closed before it can report an error.
When this error happens you may also get a cryptic 'Unknown service requested [org.hibernate.stat.spi.StatisticsImplementor]; nested exception is org.hibernate.service.UnknownServiceException: Unknown service requested [org.hibernate.stat.spi.StatisticsImplementor]' error.
To fix this error, check to see if your code works with one record first. If it works, then try to code within the looping structure again.
The CacheManager has been shut down. It can no longer be used.
This issue seems to be related to ACF 2023. I suspect that during internal Adobe testing, there is an artifact bit of code to shut the cache manager programmatically when a certain condition exists. This has been an issue with CF11 in the past and I will write an Adobe bug for this. Restarting the CF server will fix this error for a while but the issue will likely come back again if you are using ORM and caching.
The system has attempted to use an undefined value, which usually indicates a programming error, either in your code or some system code.
There are two issues that I am documenting here:
You may be using a cfquery param tag in HQL on a primary key or relationship that has a relationship. Remove the cfquery param.
Another issue is that you may have a table name and a column name that is identical. For example, when I received this error I had a Role table and a Role column. Hibernate was confused between the object and the column when the column names are identical.
The fix here is to name the column something different than the table- such as Role for the table name, and RoleName for the column name. This issue caught me several times as I typically name the column holding the entity value the same as the table name (ie table: Role, column: Role). This is not a good idea when using ORM.
Note: Null Pointers are another name for undefined values.
This could also be the result of a ColdFusion or Hibernate bug. When you try to use an HQL query that specifies another join to a different nested object and you don't specify which item to pull from the nested array, any column listed after that will raise this error message. You may need to prefix the column with the reference to the object like so: ThemeSettingRef.ThemeSettingId as ThemeSettingId
You have attempted to dereference a scalar variable of type class java.util.ArrayList as a structure with members.
You may have forgotten to add the index of the array like so:
<cfset BlogDbObj = entityLoadByPk("Comment", 1)>
Updated 5/27/2024
Related Entries
Tags
ColdFusion ORMThis entry was posted on June 8, 2022 at 7:56 PM and has received 1724 views.