Working with JSON and JavaScript
Sep 22 |
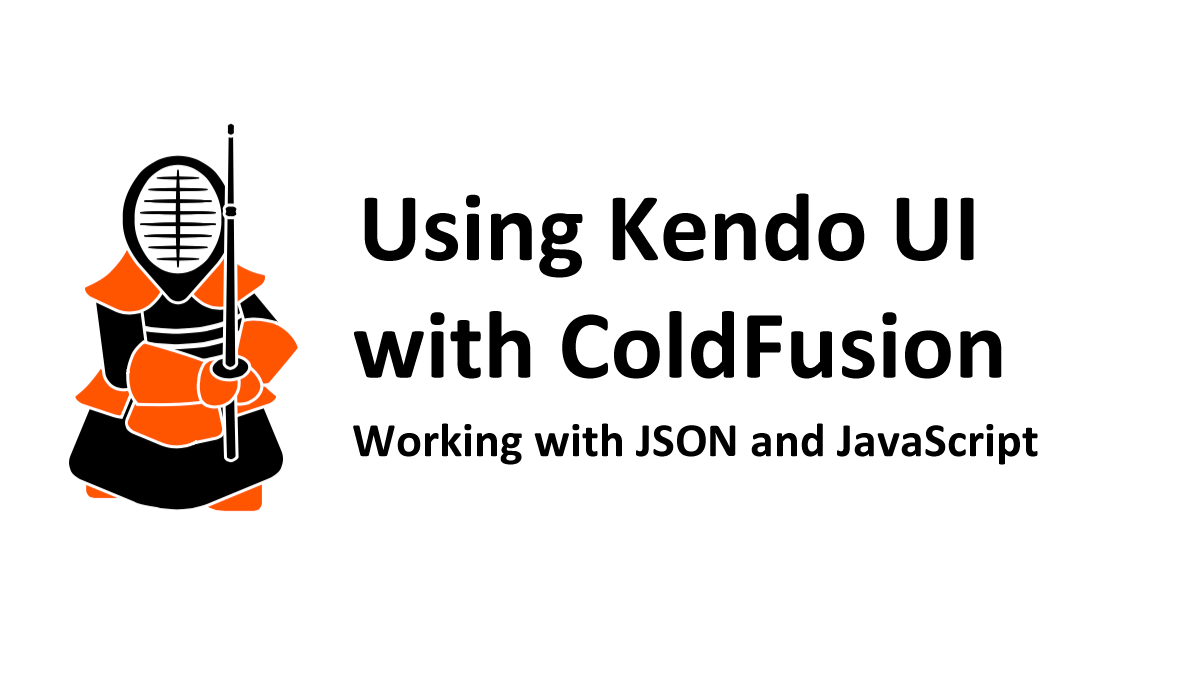
In my ColdFusion and Kendo UI articles series, I have covered how to use JSON to populate the Kendo UI widgets. However, JSON can be used for much more than that!
JSON objects can be used by nearly every modern language, whether it is C#, Java, or ColdFusion. I use JSON almost whenever I want to transfer data from the server to the client side using JavaScript.
In this article, we will take a quick break from Kendo UI and introduce you to JSON and AJAX. I will show you how to create and consume JSON, how to consume JSON on the server using AJAX, and how to work with these JSON objects with JavaScript. Like our other articles, I will provide the code and real-time examples.
Table of Contents
What is JSON?
Simply put, JSON is a popular string format for exchanging data between applications. In web applications, it is typically used to transfer data with AJAX or interact with an API.
JSON typically contains an array of structures but can also be a single double-quoted string. JSON is not inherently an object but describes object data in JavaScript and other languages. However, once JSON is successfully parsed by JavaScript (or other languages), the JSON information is transformed into a native JavaScript object.
JSON Structure
The example below is a typical JSON structure that puts the data elements within an array of structures. However, as we mentioned before, you can also use simple strings in JSON as long as double quotes enclose the string.
The following elements can be used in the JSON string:
- strings enclosed in double quotes
- numbers are not quoted
- objects, typically a structure of key pairs enclosed by braces
- arrays surrounded by square brackets.
- boolean values do not use quotes
- null uses null without quotes
See https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/JSON for more detailed information.
[
{
"Description":"All functionality.",
"RoleId":1,
"RoleName":"Administrator",
"Capabilities": ["AssetEditor",
"EditCategory",
"EditComment",
"EditFile",
"EditPage",
"EditPost",
"EditProfile",
"EditServerSetting",
"EditSubscriber",
"EditTemplate",
"EditTheme",
"EditUser",
"ReleasePost"],
"Notes":null,
"Active":true
}
]
JSON Data Handles and Other Extraneous Information
While not technically part of the JSON specification, some HTML5 widget libraries, such as jsGrid, require the JSON to have a data handle. The data handle in the example below is the "data": string.
Some widgets, including Kendo UI, also require the JSON to have a total structure in the JSON to display the total number of records in a grid for pagination purposes.
JSON is a flexible specification; as long as you follow the basic rules, this information can be incorporated. Extra information in the JSON can also be ignored.
JSON Example with a Data Handle
{
"data":[
{
"Email":"myemail@gmail.com",
"Description":"We have covered how to use JSON to populate Kendo UI objects, but JSON can do much more than that! JSON can be used in nearly every language, however, in this article, we will discuss how to work with JSON objects in JavaScript.",
"BlogSortDate":"September, 16 2022 23:32:00",
"MimeType":null,
"PostAlias":"Working-with-JSON-and-JavaScript",
"Released":false,
"Body":"In my series of ColdFusion and Kendo UI articles, I have covered how to use JSON to populate the Kendo UI widgets. However, JSON can be used for much more than that!",
"Title":"Working with JSON and JavaScript",
"NumViews":0,
"Date":"September, 20 2022 00:00:00",
"FullName":"Gregory Alexander",
}
]
}
JavaScript JSON-Related Functions
The following static JavaScript functions are used with JSON:
- JSON.parse(string);
JSON.parse is used to convert a JSON string into a JavaScript object.
See https://www.w3schools.com/js/js_json_parse.asp for more information. - JSON.stringify(string);
JSON.stringify converts a JavaScript object back into a JSON string.
See https://www.w3schools.com/js/js_json_stringify.asp for more information.
Extracting Data from a JSON String
To extract the value of a single element in the JSON string, use variableName.key. This is identical to getting a value stored in ColdFusion structures. For example, to get the post title in the JSON above, use data.Title
.
You can also use bracket notation, just as you would get the value of a ColdFusion HQL column using variableName["key"]. Using this notation, we would use data["Title"]
. Both of these statements will do the same thing.
A Brief Introduction to AJAX and JSON
There are many other ways to elicit a JSON response from the server. Still, this article will focus on using AJAX on the client side to send an asynchronous HTTP (Ajax) request to a ColdFusion Component or function. The ColdFusion template on the server will process the data and send a JSON response to the calling AJAX function. We will then inspect the AJAX response and deliver information back to the user using JavaScript.
This AJAX request may be made by a traditional AJAX statement or performed automatically using the Kendo DataSource when we declare a Kendo DataSource or initialize a Kendo UI widget.
AJAX Automatically Transforms JSON into JavaScript
It is important to note that if you use AJAX to consume JSON on the server, the JSON string will automatically be converted into a native JavaScript object when using "json" as the dataType argument. After transforming the JSON string, you must use native JavaScript methods to get at the underlying data in the JavaScript object.
What is the Difference Between AJAX and getJSON?
You may either use jQueries AJAX or the getJSON method to fetch JSON data using a get HTTP request. The getJSON function is a simplified version of jQuery's AJAX; however, the two methods are the same underneath the hood.
I prefer using the AJAX method as it provides more customization; in particular, I can either enable or disable caching. Technically, there are ways to disable caching using the getJSON function. Still, they either affect all of the AJAX statements with a global AJAX cache var, or they use a timestamp method, which I find to be a bit kludgy.
The arguments for the getJSON function are:
$.getJSON(url, data, success);
Parsing JSON that has been Transformed into a JavaScript Object
There are multiple ways to get the JSON keys and values in a JavaScript object. Your approach will differ depending on whether you want to extract a single value or multiple records.
Extracting a Single Value
If you're using a single row of data or know the index of the row you want to extract, you can use data[index].key
For example, to get the state name in our result, use data[0].name. JavaScript arrays start at zero, so the first row uses an index of 0. This is identical to the approach used to parse a JSON string, although using JavaScript requires specifying an index to get the proper row.
Like JSON, you can also use the bracket notation like so:
alert(data[0]['name'])
Here is a complete example of the AJAX call:
jQuery.ajax({
type: 'post',
url: '<cfoutput>#application.baseUrl#</cfoutput>/demo/WorldCountries.cfc',
data: { // method and the arguments
method: "getStates",
state: "Washington"
},//..data: {
dataType: "json",
success: result, // calls the result function.
// Simplified error handling
error: function(ErrorMsg) {
console.log('Error' + ErrorMsg);
}
});//..jQuery.ajax({
function result(data){
alert(data[0].name);
}
Looping Through Multiple Records
You can loop through the JSON and retrieve an item using the following script if you have multiple records. I am using bracket notation in this script.
for(var i=0; i < data.length; i++){
// Get the data held in the row in the array using bracket notation
alert(data[i]['name'])
}
A complete example with an HTML form populated by AJAX and JSON. A real-time example is provided below.
<script>
// Get state data from the server
jQuery.ajax({
type: 'post',
url: '<cfoutput>#application.baseUrl#</cfoutput>/demo/WorldCountries.cfc',
data: { // method and the arguments
method: "getStates",
countryId: 233 //United States
},//..data: {
dataType: "json",
success: result, // calls the result function.
// Simplified error handling
error: function(ErrorMsg) {
console.log('Error' + ErrorMsg);
}
});//..jQuery.ajax({
function result(data){
for(var i=0; i < data.length; i++){
// Get the name of the state held in the row in the array and push it to the form
$("#state" + i).val(data[i]['name']);
}//for(var i=0; i < result.data.length; i++){..
}//function result(data){..
</script>
<p>This form is populated by AJAX and JSON</p>
<table width="100%" class="k-content">
<!--- Loop 66 times, some states are territories here --->
<cfloop from="0" to="65" index="i">
<tr>
<td align="left" valign="top" class="border" colspan="2"></td>
</tr>
<tr>
<td align="right" style="width: 20%">
<label for="state<cfoutput>##</cfoutput>">State:</label>
</td>
<td>
<!-- Create the state text input -->
<input type="text" id="state<cfoutput>#i#</cfoutput>" name="state<cfoutput>#i#</cfoutput>" value="" style="width: 95%">
</td>
</tr>
</cfloop>
<tr>
<td align="left" valign="top" class="border" colspan="2"></td>
</tr>
</table>
Inspecting the Entire Object
Finally, you may inspect the entire object using JavaScript like so:
function result(data){
// Loop thru the outer object (data)
for(var i=0; i < data.length; i++){
// Get the data held in the row in the array.
var obj = data[i];
// Create an inner for loop
for(var key in obj){
// Set the values.
var attrName = key;
var attrValue = obj[key];
alert(attrName);
alert(attrValue);
}
}
}
Complete Example with AJAX:
<script>
// Get state data from the server
jQuery.ajax({
type: 'post',
url: '<cfoutput>#application.baseUrl#</cfoutput>/demo/WorldCountries.cfc',
data: { // method and the arguments
method: "getStates",
state: "Washington"
},//..data: {
dataType: "json",
success: result, // calls the result function.
// Simplified error handling
error: function(ErrorMsg) {
console.log('Error' + ErrorMsg);
}
});//..jQuery.ajax({
function result(data){
// Loop thru the outer object (data)
for(var i=0; i < data.length; i++){
// Get the data held in the row in the array.
var obj = data[i];
// Create an inner for loop
for(var key in obj){
// Set the values.
var attrName = key;
var attrValue = obj[key];
// Pop the values up
alert('Attribute Name: ' + attrName);
alert('Attribute Value: ' + attrValue);
}//for(var key in obj){..
}//for(var i=0; i < data.length; i++){..
}//function result(data){..
</script>
This page retrieves the state using AJAX and will pop up an alert showing the keys and values found in the object. There should be 8 popups here.
Our next article will use some of what we learned here today.
Further Reading
- jQuery AJAX (jQuery)
- jQuery getJSON (jQuery)
- Working with JSON (MDN Documentation)
- JSON Datatypes (W3Schools.com)
Related Entries
Tags
JSON, JSON
![]() |
Gregory Alexander |
Hi, my name is Gregory! I have several degrees in computer graphics and multimedia authoring, and I have been developing enterprise web applications for the last 25 years. I love web technologies and the outdoors and am passionate about giving back to the community. |
This entry was posted on September 22, 2022 at 11:22 PM and has received 1818 views.