Introducing the various forms of Kendo Dropdowns
Sep 7 |
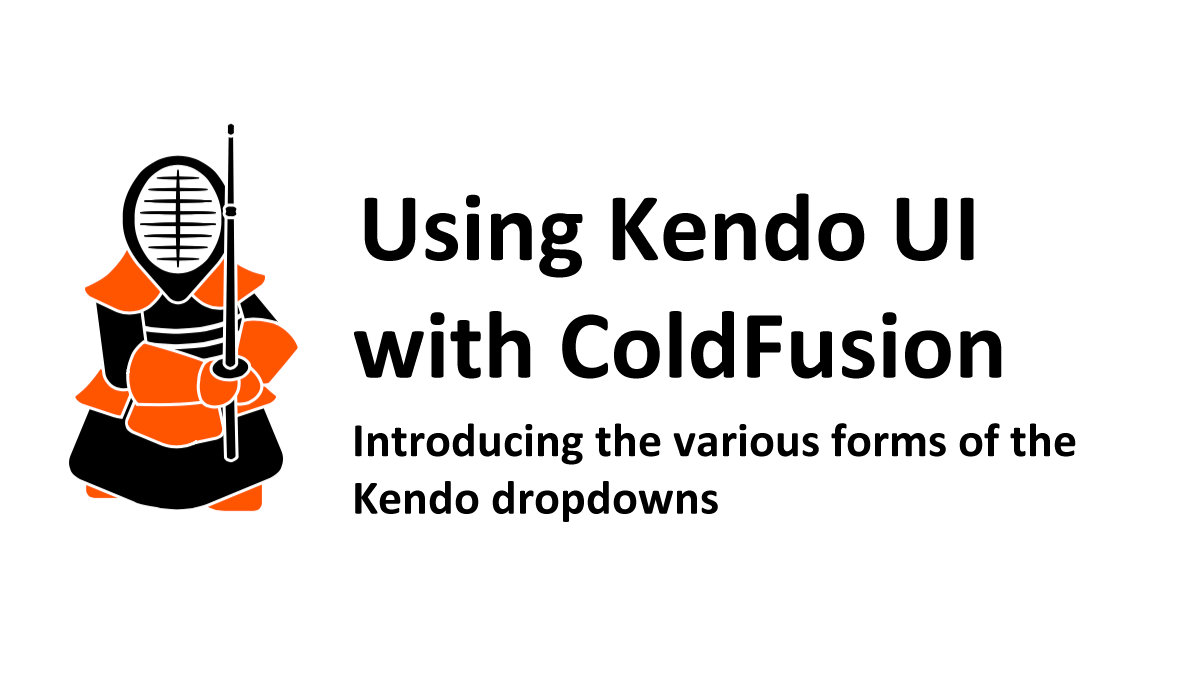
There are three major Kendo Dropdowns: the Kendo DropDown List, ComboBox, and Multi-Select. All of these Kendo widgets enhance the traditional HTML select input and add rich HTML5 functionality.
However, unlike a traditional HTML dropdown, it offers several features, such as having a search input at the top of the menu to allow users to find their intended choice quickly. This can save the end-users time and agony when the dropdown contains a list of choices, as the user can quickly type in a value in the search field and select the intended choice. I have often wished that other sites had this capability when asked to select a value from a long list of choices, such as choosing my country and state.
Additionally, these drop-downs support sophisticated data operations, such as virtualization and grouping, and these elegant drop-downs take on the characteristics of your chosen theme. You can apply Kendo Templates to the drop-downs to extend their functionality, such as adding images to the drop-down choices.
These controls can be data bound to a traditional select input using option tags, a local JavaScript array, or a remote data service using a server-side language such as ColdFusion. Refer to our previous Using ColdFusion to Populate Kendo UI Widgets for in-depth examples of each option. If you want an example of how to bind the widgets to a local JavaScript array, see Using ColdFusion to Synchronize Time Across Time Zones.
In this article, we will discuss the differences between these elements and show examples of each dropdown type that binds the Kendo DataSource to a server-side end-point using ColdFusion.
Table of Contents
The Kendo DropDownList
In its most basic form, the Kendo DropDown List replicates the normal HTML dropdown in that the user can only choose among predetermined values.
This dropdown can be created manually by creating your option tags in HTML, bound to data using JavaScript arrays, or populated by a remote service.
The Kendo ComboBox
The Kendo ComboBox is identical to the Kendo DropDownList with just one main difference- the ComboBox also allows the user to enter a custom value in the search bar. If the value does not match one of the values, the ComboBox allows you to launch a new interface to allow the user to enter the new value.
To put it another way, a Combo Box is often termed an editable dropdown list, whereas a Dropdown list is a non-editable dropdown list. For more information on the Combo Box across various platforms, see https://en.wikipedia.org/wiki/Combo_box.
To illustrate, in the example below, I developed an interface to allow the administrators to specify fonts for unique portions of the page; however, if the user types in a font that does not exist in the search bar at the top of the dropdown widget, the user is also able to upload a new font and have it displayed in the Kendo ComboBox.
Kendo Multi-Select
Unlike the dropdown list and ComboBox widgets, the Kendo Multi-Select is a unique dropdown widget that allows the user to select multiple values from a dropdown list. This sophisticated widget can replace a long list of checkboxes on a form, allowing the user interface to be streamlined.
Like the DropDownList and ComboBox, this widget also has search capabilities, allowing users to select multiple values quickly. I use this widget on the blog to allow the user to choose specific categories when performing a site search. The Kendo MultiSelect component dramatically simplifies the Galaxie Blog search interface- without this component, I would have to loop through every category and build checkboxes, making the interface very long and confusing.
Similarities and Differences Between The Kendo Dropdowns
As we just mentioned, if appropriately configured with the filter argument, the Kendo DropdownList and ComboBox offer nearly identical functionality. Almost all of the code is interchangeable between the two widgets.
In fact, in the ComboBox example below, you can change this widget to a dropdown list by changing the first line of the code below by substituting the string 'kendoComboBox' with 'kendoDropDownList'. However, changing the widget to a Kendo Dropdown list will not add a new item to the list when you enter a value using the search function that is not found.
Remember that the DropDownList may also be considered a non-editable dropdown, whereas the ComboBox is an editable dropdown. In Kendo UI's implementation, this means that if the search term is not found, you can add a new item to the list (and optionally to the database) with a new interface.
You may also replicate the functionality of the ComboBox with the DropDownList, but it will require extra code that causes the dropdown to refresh if a new item has been added using the noDataTemplate, which we will cover later. I would not be surprised to see Telerik consolidate the two widgets eventually.
The purpose of the Kendo MultiSelect widget is a different matter- it is meant to allow the user to select multiple values using a familiar dropdown interface. As we have already covered, this widget can also replace a long list of checkboxes, making it easier for the user to select an option, and it clears up valuable real estate on the screen.
The code used to initialize all of these widgets is nearly identical. You can usually substitute one for another by changing a couple of lines of code. There are some other minor differences. However, placeHolder is used for the ComboBox and MultiSelect, and optionLabel is used for the dropdown list to make an inline hint when nothing is selected, for example.
We will cover the widget's basic initialization below.
ComboBox Javascript Initialization
The only difference between this and the code below is the string kendoComboBox on the first and last lines and placeHolder in the second line. The rest of the code is identical between the two widgets.
var blogNameFontDropdown = $("#blogNameFontDropdown").kendoComboBox({
placeHolder: "Select...",
autoBind: false,
dataTextField: "Font",
dataValueField: "FontId",
template: '<label style="font-family:#:data.FontFace#">#:data.Font#</label>',
// Template to add a new type when no data was found.
noDataTemplate: $("#addFont").html(),
filter: "contains",
dataSource: fontDs,
}).data("kendoDropDownList");
See https://demos.telerik.com/kendo-ui/combobox/index for more information.
DropDownList Javascript Initialization
The only difference between this and the code below is the string kendoDropDownList on the first and last line and optionLabel in the second line.
var blogNameFontDropdown = $("#blogNameFontDropdown").kendoDropDownList({
optionLabel: "Select...",
autoBind: false,
dataTextField: "Font",
dataValueField: "FontId",
template: '<label style="font-family:#:data.FontFace#">#:data.Font#</label>',
// Template to add a new type when no data was found.
noDataTemplate: $("#addFont").html(),
filter: "contains",
dataSource: fontDs,
}).data("kendoDropDownList");
See https://demos.telerik.com/kendo-ui/dropdownlist/index for more information.
Kendo MultiSelect Javascript Initialization
The line to initialize the multi-select is kendoMultiSelect, and like the Kendo ComboBox, this uses the placeholder argument to specify a hint when nothing is selected.
var blogNameFontDropdown = $("#blogNameFontDropdown").kendoMultiSelect({
placeholder: "Select...",
autoBind: false,
dataTextField: "Font",
dataValueField: "FontId",
template: '<label style="font-family:#:data.FontFace#">#:data.Font#</label>',
// Template to add a new type when no data was found.
noDataTemplate: $("#addFont").html(),
filter: "contains",
dataSource: fontDs,
}).data("kendoMultiSelect");
See https://demos.telerik.com/kendo-ui/multiselect/index for more information.
The Kendo DataSource Used for these Widgets
This particular data source acquires the data remotely using a get method from the ProxyController.cfc ColdFusion component and returns the data as a JSON object. It should be noted that all of the widget examples use the same fonts as the Kendo data source and have no code changes.
As stated in other articles, you can use the Kendo Datasource inline within the widgets or create a unique data source detached from the widget. I typically detach the Kendo DataSource to reuse it, as we have done here. The data source can be reused for nearly all kendo widgets, even the grid. In the future, I plan on writing new articles on the Kendo Grid and will reuse the same Kendo Datasource I have used here.
Here is the fontDs Kendo DataSource Javascript initialization that is used for all of these examples:
var fontDs = new kendo.data.DataSource({
transport: {
read: {
cache: false,
// Note: since this template is in a different directory, we can't specify the cfc template without the full path name.
url: function() { // The cfc component which processes the query and returns a json string.
return "<cfoutput>#application.baseUrl#</cfoutput>/common/cfc/ProxyController.cfc?method=getFontsForDropdown";
},
dataType: "json",
contentType: "application/json; charset=utf-8", // Note: when posting json via the request body to a coldfusion page, we must use this content type or we will get a 'IllegalArgumentException' on the ColdFusion processing page.
type: "GET" //Note: for large payloads coming from the server, use the get method. The post method may fail as it is less efficient.
}
} //...transport:
});//...var fontDs...
See https://docs.telerik.com/kendo-ui/api/javascript/data/datasource for more information.
The ColdFusion Remote Endpoint for the DataSource
The Kendo Datasource used for all of these widgets uses the same ColdFusion remote endpoint service provided by the ProxyController.cfc component.
If you're trying to follow along with ColdFusion, please see https://www.gregoryalexander.com/blog/2022/7/13/Using-ColdFusion-to-Populate-Kendo-UI-Widgets and download the CFJson ColdFusion component if you don't already have it. This component is necessary to convert ColdFusion objects into JSON, which the Kendo Datasource uses.
Most of this code should be self-explanatory. We are simply querying the database using ColdFusion ORM and returning a JSON object without a data handle. We can also use native ColdFusion queries using the 'convertCfQuery2JsonStruct' method in the same CF.
When using a remote service to populate Kendo controls you must use 'access="remote"' on the ColdFusion method. See https://www.gregoryalexander.com/blog/2022/7/13/Using-ColdFusion-to-Populate-Kendo-UI-Widgets for more information.
<cffunction name="getFontsForDropdown" access="remote" returnformat="json" output="false"
hint="Returns a json array to populate the font dropdown.">
<!--- Note: the csrfToken is not required for this query is also used on the external blog (non-admin). This query is not secured. --->
<!--- Get the fonts, don't use the cached values. --->
<cfquery name="Data" dbtype="hql">
SELECT DISTINCT new Map (
FontId as FontId,
Font.Font as Font
)
FROM
Font as Font
</cfquery>
<!--- Return the data as a json object. --->
<cfinvoke component="#jsonArray#" method="convertHqlQuery2JsonStruct" returnvariable="jsonString">
<cfinvokeargument name="hqlQueryObj" value="#Data#">
<cfinvokeargument name="includeTotal" value="false">
<cfinvokeargument name="includeDataHandle" value="false">
</cfinvoke>
<!--- Return the json string. --->
<cfreturn jsonString>
</cffunction>
Using Kendo UI Templates in the Dropdowns
Kendo UI Templates can be used to customize the display or extend the basic dropdown functionality. We will briefly review some of the most basic information to help you better understand how we are using templates in this article.
Kendo UI uses its proprietary syntax similar to ColdFusion, using hashes to render JavaScript variables.
Kendo UI Template Syntax
In a nutshell, the following syntax is used to render JavaScript variables:
#= javascriptVar #
Renders the value as HTML- #: javascriptVar # Uses HTML to encode JavaScript values
# if (true) { # ... non-script content here ... # } #
Conditional inline statement.
Common Dropdown Template Types
These most commonly used templates are available to the ComboBox, DropDownList, and MultiSelect:
- FooterTemplate
- Header Template
- No DataTemplate
- and more...
Look at the widgets API on the Kendo site for more information regarding the available templates.
Inline versus External Templates
A Kendo Template can either be an inline or an external template. An inline template typically has one line of code, or it can be a simple string that is used for display purposes. For example, an inline template can be as simple as this:
noDataTemplate: '<p>Font does not exist!</p>'
An external template is a JavaScript snippet wrapped with an Id that is used to specify the template in the widget's initialization script along with the type 'tex/x-kendo-template':
<script id="javascriptTemplate" type="text/x-kendo-template">
<p>Font does not exist</p>
An external template can also be stored remotely on a different file share or server, but we won't cover this here.
We will show you examples of both templates in the code below, but in general, you should use an inline template for simple display purposes and an external template for more complex interfaces.
See https://docs.telerik.com/kendo-ui/framework/templates/overview for more information.
Embedding Font Faces Inside the Dropdown Options Using an Inline Kendo Template
To show off the capabilities of the Kendo Templates, we will use the template to dynamically display the font face within all of these Kendo dropdowns. Every new option in the dropdown will display the font face. This can be done with regular HTML dropdowns, but it requires a lot of manual HTML and CSS code to fix the font for the new options in the dropdown.
With a Kendo template, we can specify all of the logic in a single line using a Kendo inline template like so:
template: '<label style="font-family:#:data.FontFace#">#:data.Font#</label>',
This particular template is used in all of the Kendo dropdown examples we have covered so far to display the proper font inside all dropdown values. However, the font display was disabled for iOS clients as more logic is necessary to load the fonts, and we don't have time to add the code now.
We will go over the details of this code later in the article.
Using the Kendo NoData Template
The Kendo No Data Template is used when there is no data or when the user types in a value not found in the dropdown. It can display feedback to the user or serve as a bridge to a different interface.
I frequently use the No Data Template in Galaxie Blog to allow users to add a new option to the dropdowns when the search term they use does not match any existing options. For example, when editing or creating a post, the user has a multi-select dropdown to assign the post to specific categories. If the category does not exist, the no data template allows the user to add a new category without exiting the post interface. The no-data template acts as a bridge to open up a new category interface, saving time for the user.
In the example below, we will use an external template instead of the inline template we used above. The template will be displayed whenever there is no data or when the search term is not found. This no-data template can be used for all of the dropdown widgets covered here, and there will be no changes to the code.
<!--- External template to add a new font. This template can be used for the ComboBox, DropDownList and MultiSelect. --->
<script id="addFont" type="text/x-kendo-tmpl">
<div>
Font not found. Do you want to add '#: instance.filterInput.val() #'?
</div>
<br />
<button class="k-button"Add Font</button>
In this example, the script Id, 'addFont', will be used to identify the template when we initialize the widget—the type 'text/x-kendo-tmpl' indicates that this is a Kendo template. The string 'instance.filterInput.val()' will display the search term used, and we are placing an HTML button to launch a new font interface at the bottom of the template. The rest of the code should be self-explanatory.
If you want to see the noData template in action, open the second button below and type 'foo' into the search control at the top of the widget. You should see an interface that provides feedback that 'foo' was not found, and the button will be displayed at the end of the interface. In this example, the button is disabled for display purposes only. The other two controls will only show that no data is available.
See https://docs.telerik.com/kendo-ui/api/javascript/ui/dropdownlist/configuration/nodatatemplate for more information.
See these Widgets in Action
In the following article, I will cover more advanced functionality of these dropdowns and show you how to use cascading dropdowns for each of these widgets.
Related Entries
Tags
ColdFusion and Kendo UI
![]() |
Gregory Alexander |
Hi, my name is Gregory! I have several degrees in computer graphics and multimedia authoring, and I have been developing enterprise web applications for the last 25 years. I love web technologies and the outdoors and am passionate about giving back to the community. |
This entry was posted on September 7, 2022 at 12:30 PM and has received 3742 views.