Using ColdFusion to Populate Kendo UI Widgets
Jul 13 |
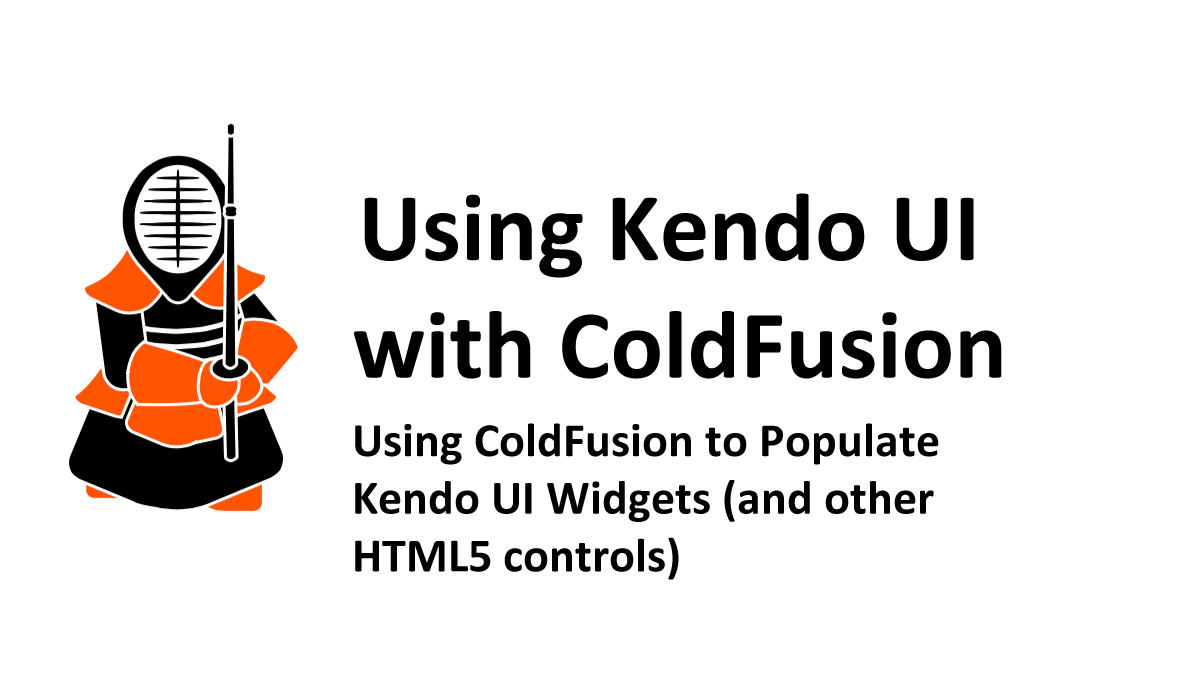
There are multiple ways to populate Kendo widgets with data. Take a simple dropdown, you can populate a dropdown the same way that you would build a simple HTML dropdown using a ColdFusion query loop or create a static dropdown by building the HTML option tags in the dropdown manually. You can also use Javascript arrays as the data source for a dropdown, or other Kendo HTML5 widgets, but to leverage the full dynamic potential of the Kendo widgets, you need to use a server-side language, such as ColdFusion, to query a database and return the data as JSON.
Table of Contents
- Populating a Kendo widget with static HTML
- Populating the Kendo DataSource with a local Javascript array
- Binding the Kendo control to a ColdFusion remote data service
- Create a Kendo DataSource and the dropdown on the client to invoke the ColdFusion service
- Further Reading
Populating a Kendo widget with static HTML
Here is an example of a simple Kendo dropdown list using static HTML:
JavaScript:
// Bind the DropDownList from static HTML
$("#serverSideLanguageDropdown").kendoDropDownList();
HTML:
<select id="serverSideLanguageDropdown">
<option value="ColdFusion">ColdFusion</option>
<option value="Lucee">Lucee</option>
</select>
Populating the Kendo DataSource with a local JavaScript array
For most widgets, you can also bind the Kendo dataSource of the widget to a local JavaScript array. This is useful if you don't have the data in the database. The arrServerSideLanguage JavaScript array is bound to the DataSource variable in the Kendo dropdown. We will cover the Kendo DataSource more extensively in the section below.
<script>
// Create a Javascript array to populate the Kendo dropdown
var arrServerSideLanguage = [
{"label":"Adobe ColdFusion","value":"ACF"},
{"label":"Lucee","value":"Lucee"}
]
// Create the Kendo dropdown
var serverSideLanguage = $("#serverSideLanguage").kendoDropDownList({
optionLabel: "Select...",// Default label
dataTextField: "label",// Dropdown label
dataValueField: "value",// Dropdown value
filter: "contains",// Search filter on the dropdown
dataSource: arrServerSideLanguage,// The datasource takes the Javascript array to populate the control
}).data("kendoDropDownList");
Binding the Kendo control to a ColdFusion remote data service
You will often bind a Kendo widget to a remote endpoint. This will be a multi-step process.
Don't worry if you don't completely understand this tutorial. This article is meant as an introduction to the process, and we will cover these steps again.
- First, we need to create a Kendo DataSource to handle our Ajax operations, which we call a ColdFusion server-side template. In this example, our service endpoint is Demo.cfc.
- Our endpoint will be a component on a ColdFusion server that retrieves data from a database and packages it into a JSON object. To do this, we need to download the CfJson component for ColdFusion.
- Once the data is prepared on the server, we will return it to the client using Ajax and pass the JSON data object to the Kendo widgets DataSource.
Create a Kendo DataSource and the dropdown on the client to invoke the ColdFusion Service
The Kendo DataSource is a component that allows you to populate the various Kendo controls with local JavaScript arrays or remote XML, JSON, or JSONP data. It also allows for server-side sorting, paging, filtering, grouping, and data aggregates.
The Kendo DataSource handles the necessary AJAX operations and posts an AJAX to the server in the URL argument below.
You will see other Kendo examples where all of the Kendo DataSource logic is embedded inside the widget. I typically separate the Kendo DataSource from the widget, as this allows me to potentially reuse the data for other controls.
<script>
// ---------------------------- Kendo datasource for the dropdown. ----------------------------
var bestLanguageDs = new kendo.data.DataSource({
transport: {
read: {
cache: false,
// Note: since this template is in a different directory, we can't specify the cfc template without the full path name.
url: function() { // The cfc component which processes the query and returns a json string.
return "<cfoutput>#application.baseUrl#</cfoutput>/demo/Demo.cfc?method=getBestLanguage";
},
dataType: "json",
contentType: "application/json; charset=utf-8", // Note: when posting json via the request body to a coldfusion page, we must use this content type or we will get a 'IllegalArgumentException' on the ColdFusion processing page.
type: "GET" //Note: for large payloads coming from the server, use the get method. The post method may fail as it is less efficient.
}
} //...transport:
});//...var bestLanguageDs...
// ---------------------------- Kendo dropdown. ----------------------------
var serverSideLanguageDropdown = $("#serverSideLanguageDropdown").kendoDropDownList({
optionLabel: "Select...",
autoBind: false,
dataTextField: "label",
dataValueField: "value",
filter: "contains",
dataSource: bestLanguageDs,
}).data("kendoDropDownList");
Download the CfJson component for ColdFusion (if you don't already have it)
To query a database and return the data as a JSON object to the client, we need to use ColdFusion on the server side with a custom CFJson component. You can download this component from GitHub at https://github.com/GregoryAlexander77/CfJson if you want to follow along.
Of course, we also need to use jQuery for the Ajax operations, but Kendo UI for jQuery requires jQuery, so this should not be an issue.
Create a server-side function to use as the ColdFusion endpoint.
This function will query the database and return the data as a JSON object to the client. The following function will be included in the demo.cfc component that I use for demonstration purposes. The function access argument must be remote when performing Ajax operations. Note the returnFormat="json" argument. This must be set to JSON. Otherwise, the function will return plain text or WDDX, and the client-side Ajax will fail.
Note: I don't exactly have a 'ServerLanguage' table anywhere in a database, so in this example, I will mimic a query object by building it in code.
<cffunction name="getBestLanguage" access="remote" returnformat="json" output="true"
hint="Returns a JSON object back to the client to populate a Kendo dropdown. This function does not take any arguments">
<!--- Create a ColdFusion query using CFScript. --->
<cfscript>
serverLanguage = queryNew("label,value","varchar,varchar",
[
{label="Adobe ColdFusion",value="ACF"},
{label="Lucee", value="Lucee"}
]);
</cfscript>
<!--- Now convert the query object into JSON using the convertCfQuery2JsonStruct in the CFJson.cfc and pass in the 'serverLanguage' query --->
<cfinvoke component="#application.cfJsonComponentPath#" method="convertCfQuery2JsonStruct" returnvariable="jsonString" >
<cfinvokeargument name="queryObj" value="#serverLanguage#">
<cfinvokeargument name="contentType" value="json">
<cfinvokeargument name="includeTotal" value="false">
<!--- Don't include the data handle for Kendo dropdowns --->
<cfinvokeargument name="includeDataHandle" value="false">
<cfinvokeargument name="dataHandleName" value="">
<!--- Set to true to force the column names into lower case. --->
<cfinvokeargument name="convertColumnNamesToLowerCase" value="false">
</cfinvoke>
<cfreturn jsonString>
</cffunction>
Further Reading
- Kendo DropDown List Basic Usage (Telerik)
- Kendo Local Data Binding (Telerik)
- Kendo DataSource Overview (Telerik)
- Kendo DataSource Basic Usage (Telerik)
- Kendo DataSource Configuration (Telerik)
Related Entries
- Incorporate Kendo UI into a ColdFusion Application
- Convert a ColdFusion Query into a JSON Object
- Introducing the various forms of Kendo Dropdowns
- Cascading Country, State and City Kendo UI Dropdowns
- Working with JSON and JavaScript
- Dynamically Populating Kendo MultiSelects
- Introducing the Kendo Grid
Tags
ColdFusion and Kendo UI, ColdFusion and Kendo UI
![]() |
Gregory Alexander |
Hi, my name is Gregory! I have several degrees in computer graphics and multimedia authoring, and I have been developing enterprise web applications for the last 25 years. I love web technologies and the outdoors and am passionate about giving back to the community. |
This entry was posted on July 13, 2022 at 10:04 PM and has received 2088 views.