Extracting Kendo Theme Color Properties
Jan 16 |
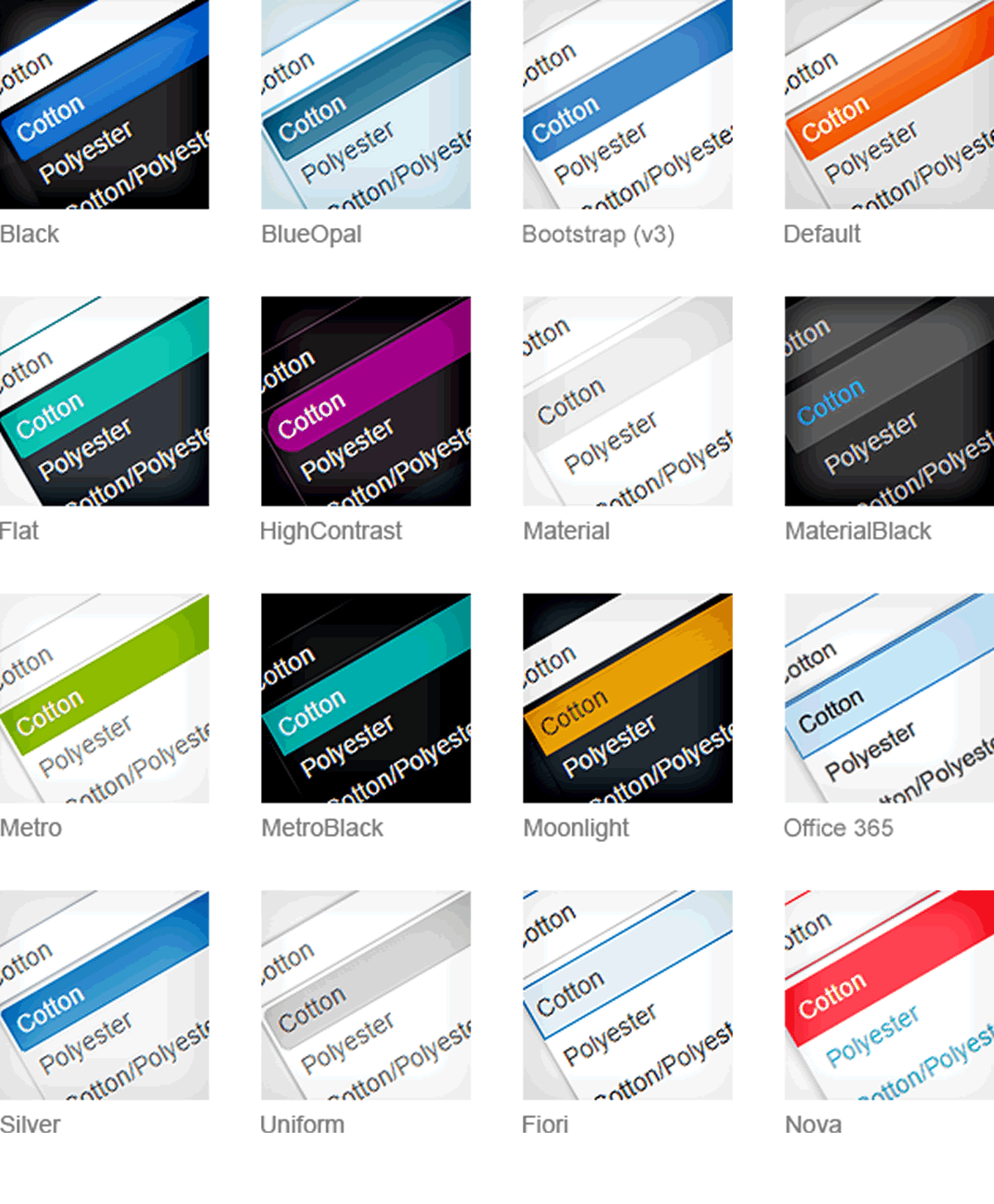
One of the advantages of using Kendo UI is that all of the theme properties of the widgets are consistently themed, however, often it is necessary to create your own custom interfaces or use other third-party libraries. There are many Kendo UI CSS classes, however, it can be difficult to figure out what each class does and there is limited documentation. In this article, I will show you how to implement a custom function to get the color properties of a selected Kendo theme and share these properties with other custom interfaces.
I developed this function using ColdFusion, however, this is essentially a list of properties and it should be easy to replicate in another language.
Table of Contents
The getPrimaryColorsByTheme Function to Extract Kendo Theme Color Properties
The ColdFusion function below extracts over a dozen different Kendo UI color properties for each less-based Kendo theme, and has two input parameters, - the Kendo Theme name and property. This function can return up to 156 unique properties.
Kendo Theme Name
This string is case-insensitive, and the supported less-based theme names are:
- black
- blueOpal
- bootStrap
- default
- flat
- highContrast
- material
- materialBlack
- metro
- moonLight
- nova
- office365
- silver
- uniform
property
There are a variety of properties that you can retrieve.The various property arguments are:
- accentColor
- baseColor
- headerBgColor
- hoverBgColor
- textColor
- selectedTextColor
- contentBgColor
- alternateBgColor
- error
- warning
- success
- info
See the examples below for more information.
Example
property | Notes | This | Kendo Class |
accentColor | corresponds to the k-primary class | ||
baseColor | corresponds to the k-widget class | ||
headerBgColor | The color of the Kendo Window title bar. Corresponds to k-header | ||
hoverBgColor | The color of the Kendo Grid cell when hovered. Corresponds to k-state-hover | ||
textColor | The font color when using the k-widget class | Text | Text |
selectedTextColor | Corresponds to the font color when the k-primary is selected. | Text | Text |
contentBgColor | Corresponds to the k-content class | ||
alternateBgColor | Corresponds to the k-alt class | ||
error | Corresponds to k-notification-error | ||
warning | Corresponds to k-notification-warning | ||
success | Corresponds to k-notification-success | ||
info | Corresponds to k-notification-info |
See https://www.gregoryalexander.com/blog/demo/kendoClasses/table.cfm to explore the properties of other Kendo themes.
Examining the Server-Side Code
The code for this function is quite straightforward. As we have covered, there are two arguments, the kendoTheme and property. The kendoTheme sets the case logic, and the property argument determines which property to extract. This particular code, minus a few modifications, has been running in my environments for several years now and it allows me to skin other user interfaces to match the chosen Kendo theme.
<cffunction name="getPrimaryColorsByTheme">
<cfargument name="kendoTheme" required="true" hint="Pass in the Kendo theme name."/>
<cfargument name="property" required="true" hint="What property name do you want to see?"/>
<cfswitch expression="#kendoTheme#">
<cfcase value="black">
<cfset buttonAccentColor = "db4240">
<cfset accentColor = "0066cc">
<cfset baseColor = "292525">
<cfset headerBgColor = "292525">
<cfset headerTextColor = "fff">
<cfset hoverBgColor = "3d3d3d">
<cfset hoverBorderColor = "4d4d4d">
<cfset textColor = "ffffff">
<cfset selectedTextColor = "ffffff">
<cfset contentBgColor = "4d4d4d">
<cfset contentBorderColor = "000">
<cfset alternateBgColor = "555">
<cfset error = "db4240">
<cfset warning = "ffc000">
<cfset success = "2b893c">
<cfset info = "0066cc">
</cfcase>
<cfcase value="blueOpal">
<cfset buttonAccentColor = "0066cc">
<cfset accentColor = "326891">
<cfset baseColor = "fff">
<cfset headerBgColor = "E3EFF7">
<cfset headerTextColor = "000">
<cfset hoverBgColor = "A1D6F7">
<cfset hoverBorderColor = "3d3d3d">
<cfset textColor = "000">
<cfset selectedTextColor = "fff">
<cfset contentBgColor = "fff">
<cfset contentBorderColor = "a3d0e4">
<cfset alternateBgColor = "e6f2f8">
<cfset error = "db4240">
<cfset warning = "ffb400">
<cfset success = "37b400">
<cfset info = "0066cc">
</cfcase>
<cfcase value="bootstrap">
<cfset buttonAccentColor = "428bca">
<cfset accentColor = "428bca">
<cfset baseColor = "fff">
<cfset headerBgColor = "f5f5f5">
<cfset headerTextColor = "333333">
<cfset hoverBgColor = "ebebeb">
<cfset hoverBorderColor = "333333">
<cfset textColor = "333333">
<cfset selectedTextColor = "fff">
<cfset contentBgColor = "fff">
<cfset contentBorderColor = "dfdfdf">
<cfset alternateBgColor = "f5f5f5">
<cfset error = "ffe0d9">
<cfset warning = "fbeed5">
<cfset success = "eaf7ec">
<cfset info = "e5f5fa">
</cfcase>
<cfcase value="default">
<cfset buttonAccentColor = "0066cc">
<cfset accentColor = "f35800">
<cfset baseColor = "fff">
<cfset headerBgColor = "eae8e8">
<cfset headerTextColor = "000">
<cfset hoverBgColor = "bcb4b0">
<cfset hoverBorderColor = "3d3d3d">
<cfset textColor = "000">
<cfset selectedTextColor = "fff">
<cfset contentBgColor = "fff">
<cfset contentBorderColor = "d5d5d5">
<cfset alternateBgColor = "f1f1f1">
<cfset error = "db4240">
<cfset warning = "ffc000">
<cfset success = "37b400">
<cfset info = "0066cc">
</cfcase>
<cfcase value="flat">
<cfset buttonAccentColor = "0066cc">
<cfset accentColor = "10c4b2">
<cfset baseColor = "fff">
<cfset headerBgColor = "363940">
<cfset headerTextColor = "fff">
<cfset hoverBgColor = "2eb3a6">
<cfset hoverBorderColor = "3d3d3d">
<cfset textColor = "000">
<cfset selectedTextColor = "fff">
<cfset contentBgColor = "fff">
<cfset contentBorderColor = "fff">
<cfset alternateBgColor = "f5f5f5">
<cfset error = "ffdfd7">
<cfset warning = "fff4d7">
<cfset success = "eefbf0">
<cfset info = "e6f9ff">
</cfcase>
<cfcase value="highContrast">
<cfset buttonAccentColor = "0066cc">
<cfset accentColor = "870074">
<cfset baseColor = "2B232B">
<cfset headerBgColor = "2c232b">
<cfset headerTextColor = "fff">
<cfset hoverBgColor = "a7008f">
<cfset hoverBorderColor = "3d3d3d">
<cfset textColor = "ffffff">
<cfset selectedTextColor = "fff">
<cfset contentBgColor = "2c232b">
<cfset contentBorderColor = "674c63">
<cfset alternateBgColor = "2c232b">
<cfset error = "be5138">
<cfset warning = "e9a71d">
<cfset success = "2b893c">
<cfset info = "007da7">
</cfcase>
<cfcase value="material">
<cfset buttonAccentColor = "0066cc">
<cfset accentColor = "5e6cbf">
<cfset baseColor = "fff">
<cfset headerBgColor = "fafafa">
<cfset headerTextColor = "000">
<cfset hoverBgColor = "ebebeb">
<cfset hoverBorderColor = "3d3d3d">
<cfset textColor = "000">
<cfset selectedTextColor = "fff">
<cfset contentBgColor = "fff">
<cfset contentBorderColor = "e6e6e6">
<cfset alternateBgColor = "F2F2F1">
<cfset error = "ffcdd2">
<cfset warning = "fdefba">
<cfset success = "c8e6c9">
<cfset info = "bbdefb">
</cfcase>
<cfcase value="materialBlack">
<cfset buttonAccentColor = "0066cc">
<cfset accentColor = "3f51b5">
<cfset baseColor = "363636">
<cfset headerBgColor = "5a5a5a">
<cfset headerTextColor = "fff">
<cfset hoverBgColor = "606060">
<cfset hoverBorderColor = "3d3d3d">
<cfset textColor = "fff">
<cfset selectedTextColor = "fff">
<cfset contentBgColor = "363636">
<cfset contentBorderColor = "4d4d4d">
<cfset alternateBgColor = "5a5a5a">
<cfset error = "c93b31">
<cfset warning = "cdaa1d">
<cfset success = "429246">
<cfset info = "207ec8">
</cfcase>
<cfcase value="metro">
<cfset buttonAccentColor = "0066cc">
<cfset accentColor = "7ea700">
<cfset baseColor = "fff">
<cfset headerBgColor = "fff">
<cfset headerTextColor = "000">
<cfset hoverBgColor = "8ebc00">
<cfset hoverBorderColor = "3d3d3d">
<cfset textColor = "000">
<cfset selectedTextColor = "fff">
<cfset contentBgColor = "fff">
<cfset contentBorderColor = "dbdbdb">
<cfset alternateBgColor = "f5f5f5">
<cfset error = "ffb8a9">
<cfset warning = "ffe44d">
<cfset success = "ddffd0">
<cfset info = "d0f8ff">
</cfcase>
<cfcase value="moonlight">
<cfset buttonAccentColor = "0066cc">
<cfset accentColor = "f4af03">
<cfset baseColor = "424550">
<cfset headerBgColor = "1f2a35">
<cfset headerTextColor = "fff">
<cfset hoverBgColor = "62656F">
<cfset hoverBorderColor = "3d3d3d">
<cfset textColor = "fff">
<cfset selectedTextColor = "000">
<cfset contentBgColor = "484c58">
<cfset contentBorderColor = "232d36">
<cfset alternateBgColor = "484c58">
<cfset error = "be5138">
<cfset warning = "ea9d07">
<cfset success = "2b893c">
<cfset info = "0c779b">
</cfcase>
<cfcase value="nova">
<cfset buttonAccentColor = "e51a5f">
<cfset accentColor = "ff5763">
<cfset baseColor = "fafafa">
<cfset headerBgColor = "fafafa">
<cfset headerTextColor = "000">
<cfset hoverBgColor = "f5f6f6">
<cfset hoverBorderColor = "FAFAFA">
<cfset textColor = "000">
<cfset selectedTextColor = "fff">
<cfset contentBgColor = "fff">
<cfset contentBorderColor = "FAFAFA">
<cfset alternateBgColor = "fafafa">
<cfset error = "ffbfc4">
<cfset warning = "ffecc7">
<cfset success = "a5d6a7">
<cfset info = "80deea">
</cfcase>
<cfcase value="office365">
<cfset buttonAccentColor = "0066cc">
<cfset accentColor = "005b9d">
<cfset baseColor = "fff">
<cfset headerBgColor = "fff">
<cfset headerTextColor = "000">
<cfset hoverBgColor = "f4f4f4">
<cfset hoverBorderColor = "c9c9c9">
<cfset textColor = "000">
<cfset selectedTextColor = "fff">
<cfset contentBgColor = "fff">
<cfset contentBorderColor = "ffff0">
<cfset alternateBgColor = "fff">
<cfset error = "fccbc7">
<cfset warning = "fff19d">
<cfset success = "cbe9cc">
<cfset info = "bbd9f7">
</cfcase>
<cfcase value="silver">
<cfset buttonAccentColor = "0066cc">
<cfset accentColor = "1984c8">
<cfset baseColor = "fff">
<cfset headerBgColor = "f3f3f4">
<cfset headerTextColor = "000">
<cfset hoverBgColor = "b6bdca">
<cfset hoverBorderColor = "F6F6F6">
<cfset textColor = "000">
<cfset selectedTextColor = "fff">
<cfset contentBgColor = "fff">
<cfset contentBorderColor = "dedee0">
<cfset alternateBgColor = "fbfbfb">
<cfset error = "d92800">
<cfset warning = "ff9800">
<cfset success = "3ea44e">
<cfset info = "2498bc">
</cfcase>
<cfcase value="uniform">
<cfset buttonAccentColor = "0066cc">
<cfset accentColor = "747474">
<cfset baseColor = "fff">
<cfset headerBgColor = "f5f5f5">
<cfset headerTextColor = "000">
<cfset hoverBgColor = "F6F6F6">
<cfset hoverBorderColor = "F6F6F6">
<cfset textColor = "000">
<cfset selectedTextColor = "FFF">
<cfset contentBgColor = "fff">
<cfset contentBorderColor = "dedee0">
<cfset alternateBgColor = "f5f5f5">
<cfset error = "d92800">
<cfset warning = "ff9800">
<cfset success = "3ea44e">
<cfset info = "2498bc">
</cfcase>
</cfswitch>
<!--- Return the property that was requested --->
<cfreturn evaluate("#arguments.property#")>
</cffunction>
Code to Invoke the Function
Like the function, the code to invoke the function is quite straightforward. Here we are setting the kendoTheme string and invoking the function and passing the kendoTheme string (in this case, 'default'), along with the property that we want to obtain.
<!--- Set the Kendo Theme var --->
<cfset kendoTheme = "default'>
<!--- Get Kendo Theme color properties --->
<cfset accentColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'accentColor')>
<cfset baseColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'baseColor')>
<cfset headerBgColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'headerBgColor')>
<cfset headerTextColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'headerTextColor')>
<cfset hoverBgColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'hoverBgColor')>
<!--- This is the separater color on the standard breadcrumb --->
<cfset hoverBorderColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'hoverBorderColor')>
<cfset textColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'textColor')>
<cfset selectedTextColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'selectedTextColor')>
<cfset contentBorderColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'contentBorderColor')>
<cfset contentBgColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'contentBgColor')>
<cfset alternateBgColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'alternateBgColor')>
<cfset errorColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'error')>
<cfset warningColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'warning')>
<cfset successColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'success')>
<cfset infoColor = getPrimaryColorsByTheme(kendoTheme:kendoTheme,property:'info')>
Code to Set CSS Variables
Finally, we are simply setting CSS variables and populating the values. We will use these CSS classes to highlight our HTML elements.
/* Kendo Theme Color Properties */
.kendo-accent-color {background-color: #<cfoutput>#accentColor#</cfoutput>; }
.kendo-base-color {background-color: #<cfoutput>#baseColor#</cfoutput>; }
.kendo-header-bg-color {background-color: #<cfoutput>#headerBgColor#</cfoutput>; }
.kendo-header-text-color {background-color: #<cfoutput>#headerTextColor#</cfoutput>; }
.kendo-hover-bg-color {background-color: #<cfoutput>#hoverBgColor#</cfoutput>; }
.kendo-text-color {color: #<cfoutput>#textColor#</cfoutput>; }
.kendo-selected-text-color {color: #<cfoutput>#selectedTextColor#</cfoutput>; }
.kendo-content-bg-color {background-color: #<cfoutput>#contentBgColor#</cfoutput>; }
.kendo-alternate-bg-color {background-color: #<cfoutput>#alternateBgColor#</cfoutput>; }
.kendo-error-color {background-color: #<cfoutput>#errorColor#</cfoutput>; }
.kendo-warning-color {background-color: #<cfoutput>#warningColor#</cfoutput>; }
.kendo-success-color {background-color: #<cfoutput>#successColor#</cfoutput>; }
.kendo-info-color {background-color: #<cfoutput>#infoColor#</cfoutput>; }
Methodology and Approach
If you want to use this approach to extract other Kendo-UI-related theme properties, determine the Kendo class that you want to use, render it to a page, and simply use one of the browser inspector tools to determine the Kendo class properties.
Related Entries
This entry was posted on January 16, 2024 at 2:58 PM and has received 234 views.