Cascading Kendo MultiSelect Dropdowns
Oct 13 |
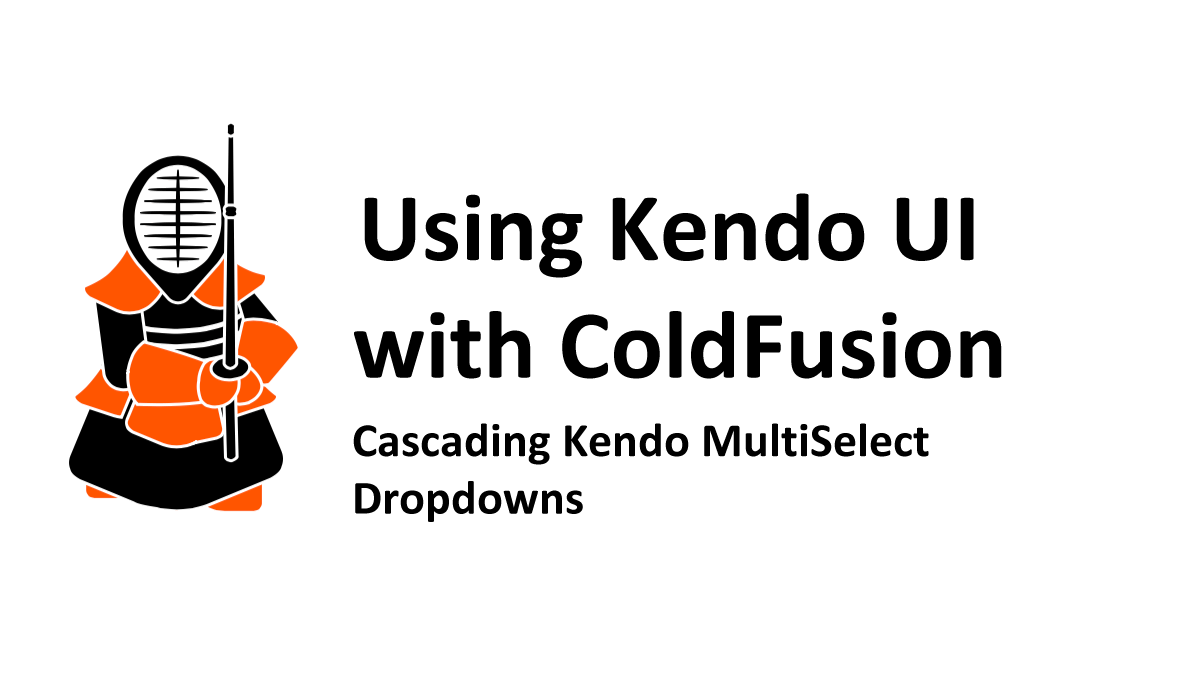
In this example, we will demonstrate how to create cascading menus' with Kendo MultiSelects. We have covered cascading menus with the dropdown lists, but there are fundamental differences.
Telerik has an example of how to create cascading dropdowns with MultiSelects, however, in my opinion, it is too complex and will suggest a simpler straightforward event-based approach. We will also briefly cover how to inspect the data coming from the parent dropdown data source and use the JavaScript push method to deselect MultiSelect values chosen by the user as well as using the Kendo DataSource to group city data in the menu.
In this example, I will try my best to replicate the UI of James Moberg's 'North America Search Demo' found at https://www.sunstarmedia.com/demo/countrystate/. James is a frequent contributor to the ColdFusion community and his elegant demo is used in production environments. Jame's demo uses a different library, Select2 UI, but it uses the same concepts that we want to show here.
Like the rest of the posts in this series, we will be using ColdFusion on the server side, however, it should be easily understood if you use a different server-side language, and you should be able to follow along.
Table of Contents
- Cascading MultiSelects Demonstration
- Cascading MultiSelects Approach
- First Country Dropdown
- Second State MultiSelect Dropdown
- The Dependent City MultiSelect Dropdown
- Client Side HTML
- Further Reading
Cascading MultiSelects Demonstration
Related Entries
Tags
ColdFusion and Kendo UI
![]() |
Gregory Alexander |
Hi, my name is Gregory! I have several degrees in computer graphics and multimedia authoring, and I have been developing enterprise web applications for the last 25 years. I love web technologies and the outdoors and am passionate about giving back to the community. |
This entry was posted on October 13, 2022 at 12:29 AM and has received 3067 views.