Incorporate Kendo UI into a ColdFusion Application
Jul 10 |
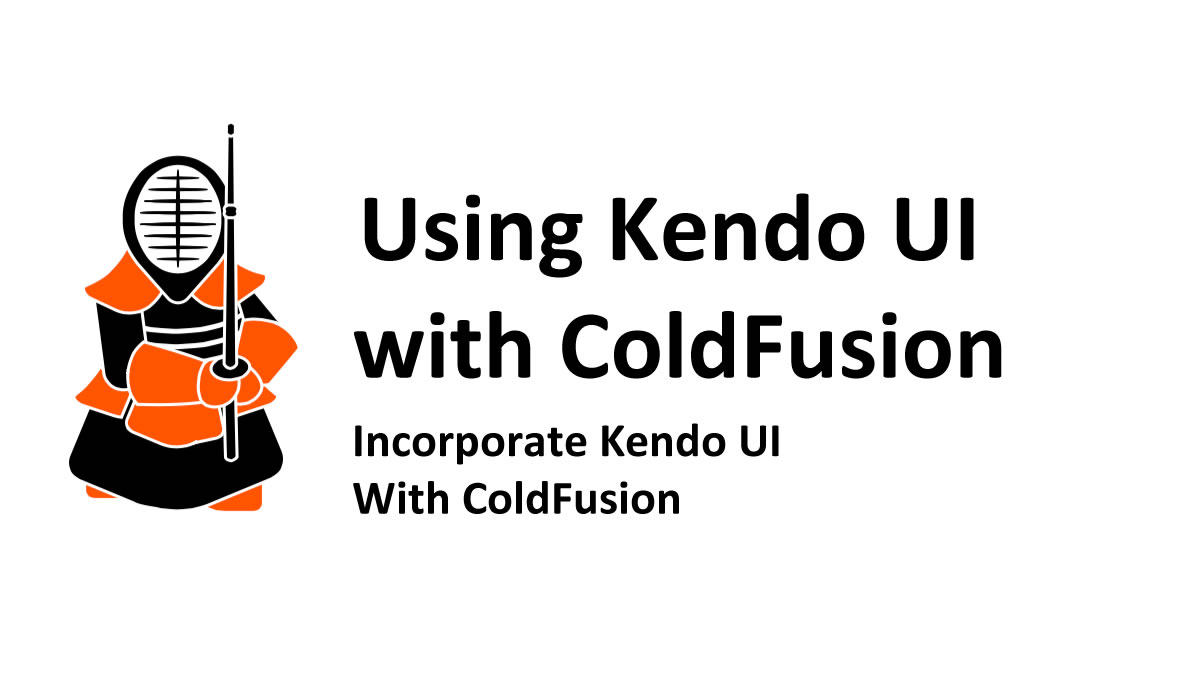
This article will help to show you what options are available when incorporating ColdFusion with Kendo UI. We will discuss the open-source and commercial versions of Kendo UI. These various sources can be used, including Kendo UI libraries with ColdFusion, and how to lazy load the library sources.
Table of Contents
- Determine if you want to use Kendo jQuery or Kendo Core
- Download the Kendo UI software or use the CDN
- Kendo Professional for jQuery Recommended Sources
- Kendo Core Recommended Sources
- jQuery Version Support
- jQuery Recommended Sources
- Add the required libraries on a web page
- Kendo UI Professional Code Examples
- Kendo Core Code Examples
- Lazy Loading Kendo UI
- Further Information
Determine if you want to use Kendo jQuery or Kendo Core
If you don't already own a Kendo license, you must determine which Kendo UI version you want to use. I have covered the different versions along with the licensing models here.
In a nutshell, if you have a large set of data that you want to share and need the power of the Kendo UI grids, you will need a professional license for Kendo for jQuery. If you don't want to spend money, are mainly interested in adding cool HTML5 widgets to an existing page, or want to open-source your project, go with Kendo Core. If you are investigating Kendo UI, you can also use professional Kendo for jQuery for 30 days.
I will cover both versions in this article.
Download the Kendo UI software or use the CDN
The developer community is divided on whether to self-host your libraries or use a Content Delivery Network (CDN).
Using a CDN is generally preferred if you have a large website with users from various locations. It is commonly accepted that you don't need a CDN if you have a website with most users from one location or a website that will not generate much traffic, such as a site running on a local intranet.
I will not wade into the details of this argument anymore, but there is another essential concern to consider if you want to use Kendo Core.
In the past, Telerik, the former company that provided Kendo, completely scrubbed its previous open-source version of Kendo UI. Unlike the current Kendo Core version, the former open-source Kendo version, Kendo UI Web Open Source, had support for the immensely popular Kendo UI grids. Telerik made a business decision to rebrand the new Kendo UI open source as Kendo Core and removed support for the grids and many other widgets. In a matter of days, it was nearly impossible to find this open-source software anymore.
My ColdFusion readers can probably relate and remember when Mura, a popular open-source CMS, suddenly eliminated all its open-source packages from the net.
If you use the CDN to host Kendo Core, download the latest version and store it as a backup. You may need it if the CDN suddenly no longer supports Kendo Core.
Kendo Professional for jQuery Recommended Sources
- Download
- CDN
- Amazon Cloud Services: https://docs.telerik.com/kendo-ui/intro/installation/cdn-service
- Kendo Static (deprecated): https://cdn.kendostatic.com/
Kendo Core Recommended Sources
- Download
- CDN
jQuery Version Support
If you are using Kendo Professional, please check the Telerik site for official jQuery support for your version at https://docs.telerik.com/kendo-ui/intro/supporting/jquery-support. However, take Teliriks default recommendations with a grain of salt.
I have noticed that Telerik's recommended default versions are quite old and have some known security issues. Most modern Kendo UI Professional versions support jQuery 3.5.1, which is secure and quite stable when used with Kendo.
jQuery is already included in the professional installation packages, so if you are already on a fast and secure private intranet, you can use the default jQuery library included in your installation. However, I would still consider upgrading your jQuery to 3.5.1.
If you are using Kendo Core, I recommend using jQuery 3.4.1. I have tested scores of jQuery versions with Kendo Core, and v3.4.1 is the latest version I could get working with Kendo Core. This is the jQuery version that I am using here with Galaxie Blog.
jQuery Recommended Sources
Regarding the CDN argument, jQuery is an entirely different beast than Kendo UI or any other software. jQuery is hosted on Google's Hosted Libraries CDN, one of the world's fastest and most geographically diverse content delivery networks. Since jQuery is one of the most widely used software libraries, with over 75% of the websites using it, your end-users likely already have a primed jQuery cached in their browsers from Google's network. Here I would strongly recommend using Google's CDN when incorporating jQuery, it will be the fastest way to deliver jQuery to the client devices, however, the choice is yours.
- Download
- jQuery site: https://jquery.com/download/
- GitHub: https://github.com/jquery/jquery
- CDN
- Google Hosted Libraries: https://developers.google.com/speed/libraries#jquery
- jQuery CDN: https://releases.jquery.com/
Add the required libraries on a web page
Once you have the required libraries in place, it is time to use them on a web page. This process is identical for all web languages, whether PHP or ColdFusion when using Kendo UI for jQuery.
- If you're self-hosting, upload Kendo UI and jQuery to your web server
- Use <!DOCTYPE html> in the very first line of the code. This will inform the browser that this page should be rendered as HTML5.
- Include Kendo UI Javascript and CSS files into the head tag of your file
- jQuery needs to be loaded before the Kendo UI script
- Place the common base Kendo UI stylesheet before the theme stylesheet.
Kendo UI Professional Code Examples
Here is a sample if you are self-hosting and using the default Kendo Professional scripts:
<!DOCTYPE html>
<head>
<!-- Load jQuery from the default Kendo installation, though you should consider using Google's CDN instead. -->
<script src="/common/libs/kendo/js/jquery.min.js"></script>
<!-- Load Kendo -->
<script src="/common/libs/kendo/js/kendo.all.min.js"></script>
<link href="/common/libs/kendo/styles/kendo.common.min.css" rel="stylesheet">
<!-- Load the Kendo theme style. -->
<link href=" /common/libs/kendo/styles/kendo.metro.min.css" rel="stylesheet">
</head>
Here is an example if you want to use one of the CDNs:
<!DOCTYPE html>
<head>
<!-- Load jQuery from Googles CDN -->
<script
src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"
crossorigin="anonymous"></script>
<!-- Load Kendo UI -->
<script src="http://kendo.cdn.telerik.com/<cfoutput>#kendoVersion#</cfoutput>/js/kendo.all.min.js"></script>
<!-- Load the stylesheets -->
<link href="http://kendo.cdn.telerik.com/<cfoutput>#kendoVersion#</cfoutput>/styles/kendo.common.min.css" rel="stylesheet" />
<link href="http://kendo.cdn.telerik.com/<cfoutput>#kendoVersion#</cfoutput>/styles/kendo.default.min.css" rel="stylesheet" />
</head>
Kendo Core Code Examples
Self Hosted
<!DOCTYPE html>
<head>
<!-- Load jQuery from Googles CDN -->
<script
src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"
crossorigin="anonymous"></script>
<!-- Load Kendo UI -->
<script src="http://kendo.cdn.telerik.com/<cfoutput>#kendoVersion#</cfoutput>/js/kendo.ui.core.min.js"></script>
<!-- Load the stylesheets -->
<link href="http://kendo.cdn.telerik.com/<cfoutput>#kendoVersion#</cfoutput>/styles/kendo.common.min.css" rel="stylesheet" />
<!-- Load the theme styles -->
<link href="http://kendo.cdn.telerik.com/<cfoutput>#kendoVersion#</cfoutput>/styles/kendo.default.min.css" rel="stylesheet" />
</head>
Kendo Core example from CDN
<!DOCTYPE html>
<head>
<!-- Load jQuery from Googles CDN -->
<script
src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"
crossorigin="anonymous"></script>
<!-- Load Kendo Core from jsdeliver.net -->
<script src="https://cdn.jsdelivr.net/npm/kendo-ui-core@2022.2.621/js/kendo.ui.core.min.js"></script>
<!-- Load the stylesheets from a local source -->
<link href="http://kendo.cdn.telerik.com/<cfoutput>#kendoVersion#</cfoutput>/styles/kendo.common.min.css" rel="stylesheet" />
<!-- Load the theme styles -->
<link href="http://kendo.cdn.telerik.com/<cfoutput>#kendoVersion#</cfoutput>/styles/kendo.default.min.css" rel="stylesheet" />
</head>
Lazy Loading Kendo UI
You can also defer these Kendo scripts to optimize the time it takes to load your page.
Please see my blog article at https://www.gregoryalexander.com/blog/2019/9/9/How-to-speed-up-your-site-with-lazy-loading
Further Information
- Telerik's First Steps article: https://docs.telerik.com/kendo-ui/intro/first-steps#1-adding-the-required-javascript-and-css-files
- Google Hosted Libraries https://developers.google.com/speed/libraries
- GitHub Kendo Core: https://github.com/telerik/kendo-ui-core
- JSDeliver Kendo Core CDN: https://www.jsdelivr.com/package/npm/kendo-ui-core
Related Entries
- How to speed up your site with lazy loading
- Benefits of ColdFusion and Kendo UI
- The Different Flavors of Kendo UI and Kendo Licensing Terms
- Convert a ColdFusion Query into a JSON Object
- Using ColdFusion to Populate Kendo UI Widgets
- A Comprehensive Look at the Kendo Window
- Introducing the various forms of Kendo Dropdowns
- Cascading Kendo MultiSelect Dropdowns
- Introducing the Kendo Grid
- A Comprehensive Analysis of the Editable Kendo UI Grid
- Implementing a Kendo Virtualized Grid with ColdFusion - Part 2, Server-Side Logic
- Dynamically Binding a Kendo Panel Bar to a Table of Contents Using jQuery
Tags
ColdFusion and Kendo UI
![]() |
Gregory Alexander |
Hi, my name is Gregory! I have several degrees in computer graphics and multimedia authoring, and I have been developing enterprise web applications for the last 25 years. I love web technologies and the outdoors and am passionate about giving back to the community. |
This entry was posted on July 10, 2022 at 3:24 PM and has received 1886 views.